Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial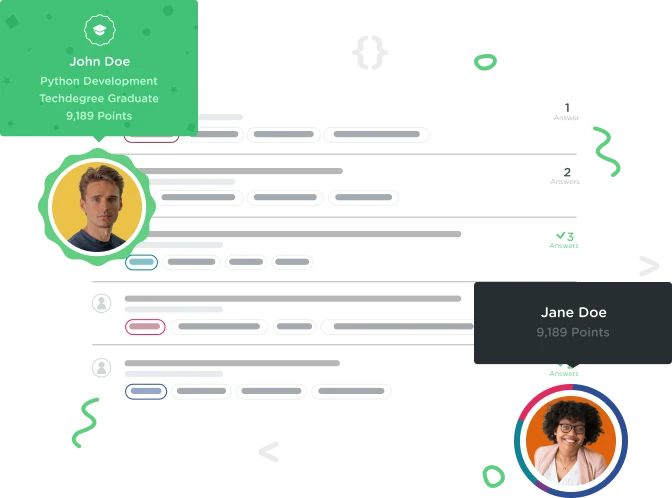
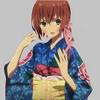
Yukiru Kannazuki
3,036 PointsHow do I assign a comma to each item in the Shopping List exercise, but add a period to the last one?
In Python's Shopping List exercise, Kenny challenges us to add a comma to the end of each item in the list, and I wanted to do something like: for item in shopping_list: while True:
if shopping_list[last]:
print(item + ". ")
break
for item in shopping_list:
print(item + ", ")
continue
I don't quite know, it still boggles my head. XD
3 Answers
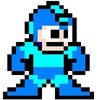
Robert Richey
Courses Plus Student 16,352 PointsHi Oak,
This is definitely a brain twister. I'll show you two ways .
# First, the hard way. Let's use a for loop to print each item
# with proper punctuation, in one line
for item in shopping_list:
if item == shopping_list[-1]:
print(item + '.')
else:
print(item + ',', end=" ")
# type help(print) to see more about the arguments into the print function
# if confused about the end keyword argument
# Next, the easy way. We can do this in just one line.
print(', '.join(shopping_list) + '.')
The output from both ways is identical.
Hope this helps,
Cheers

helmi al kindy
1,371 PointsHi Robert, Here is the code
shopping_list = list()
print("What would you like to pick up at the store ? ")
print("Enter 'DONE' to stop adding items to the list. ")
while True:
new_item = input("> ")
if new_item == 'DONE':
break
shopping_list.append(new_item)
print("Added! List has {} items.".format(len(shopping_list)))
continue
print("Here's your list: ")
for item in shopping_list:
print(', '.join(shopping_list) + '.')
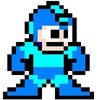
Robert Richey
Courses Plus Student 16,352 PointsThe join()
method acts on a list of values and returns a string containing all items, each separated by the given separator - in this case, the separator is the string value of a comma followed by a space.
# change this
for item in shopping_list:
print(', '.join(shopping_list) + '.')
# to this
print(', '.join(shopping_list) + '.')
# For example
print(', '.join(['apple', 'peach', 'tomato']) + '.')
# Will output: apple, peach, tomato.
# No need to use a loop here
Hope this helps!
Cheers

helmi al kindy
1,371 PointsThanks Robert it worked well.
Yukiru Kannazuki
3,036 PointsYukiru Kannazuki
3,036 PointsThanks, it was a little off from what I was thinking, but it does get what needs to be done, done. Could you explain why shopping_list[-1] works as the last index, and what end=" " does?
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsMany videos ahead of where you are, Slicing With A Step briefly explains that lists can be indexed with negative values - at about the 3:58 mark. Basically, it allows you to index into the list from the end.
The
end
keyword argument in the print function tells print what to print out last. By default, this is a new line character, but we can change this to any character we want. In this case, I changed it to a space, which allowed it to print several consecutive words on the same line.Hope this helps,
Cheers
helmi al kindy
1,371 Pointshelmi al kindy
1,371 PointsHi Robert, I did your easier method of adding comas and a dot at the end. The problem is that it prints out the list the amount of times I have added to the list instead of once. Eg: if I add 4 items to the list it will print the list 4 times instead of 1. How do I make the list print only once with the comas and dot at the end ?
Robert Richey
Courses Plus Student 16,352 PointsRobert Richey
Courses Plus Student 16,352 PointsHi Helmi,
It sounds like you may have
print(', '.join(shopping_list) + '.')
inside a loop. If it's not in a loop, I'll need to see your code to try and figure out what's happening.Cheers