Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial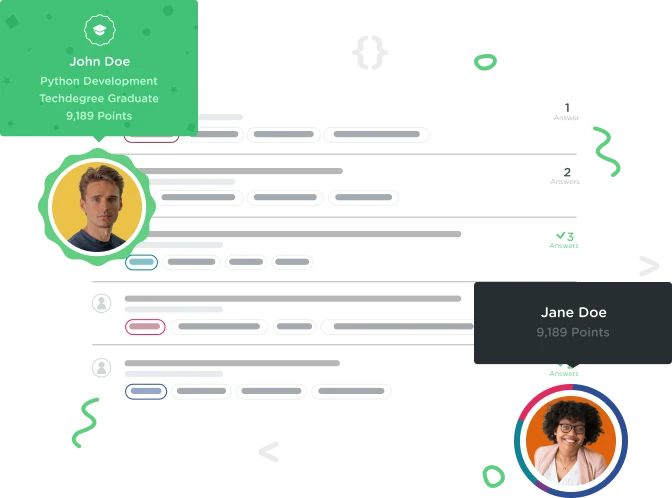
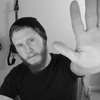
Jeremiah Shore
31,168 PointsHow do I avoid using "non-default constructors in fragments"?
I added a non-default constructor to my AlertDialogFragment class so that I can pass in the strings that will be used for the dialog. This constructor then sets the member variables, which are used in the builder.set[property] methods.
public AlertDialogFragment(String errorTitle, String errorMessage,
String errorButtonText){
mErrorTitle = errorTitle;
mErrorMessage = errorMessage;
mErrorButtonText = errorButtonText;
}
I am getting a warning from Android studio: "Avoid non-default constructors in fragments: use a default constructor plus Fragment#setArguments(Bundle) instead".
What does this mean and what would be the "proper" way to accomplish what I want to do?
2 Answers

Ken Alger
Treehouse TeacherJeremiah;
Take a look at this post over at StackOverflow. There are some great comments and examples there.
Post back with further questions.
Ken
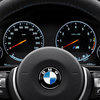
Ashley Ewen
Courses Plus Student 1,194 PointsThanks for this, I was literally having the same issue trying to reuse a alert class and wasn't sure what was going wrong.
Jeremiah Shore
31,168 PointsJeremiah Shore
31,168 PointsThanks for the direction. Looks like the most sensible answer on there is the on that starts with "Your Fragment shouldn't have constructors because of how the FragmentManager instantiates it. You should have a newInstance() static method defined and pass any parameters via arguments (bundle)", even if it isn't top voted.
Here is my re-factored code for anyone who may happen upon this question:
Here is an example of using the .newInstance method to create a new AlertDialogFragment:
Nathan Beauchamp
2,456 PointsNathan Beauchamp
2,456 PointsSo, if you use this way to customize the text in the alert dialog fragment, will you have to use the .newInstance() method every time you call it? Including our method in MainActivity alertUserAboutError()?
Ivan Sued
5,969 PointsIvan Sued
5,969 PointsJeremiah and Ken,
Thanks for the resource. I was having issues with this as well. I thought the bundle was supposed to be created in the main activity you were working with. The whole instance is what I missed. Thanks again.