Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial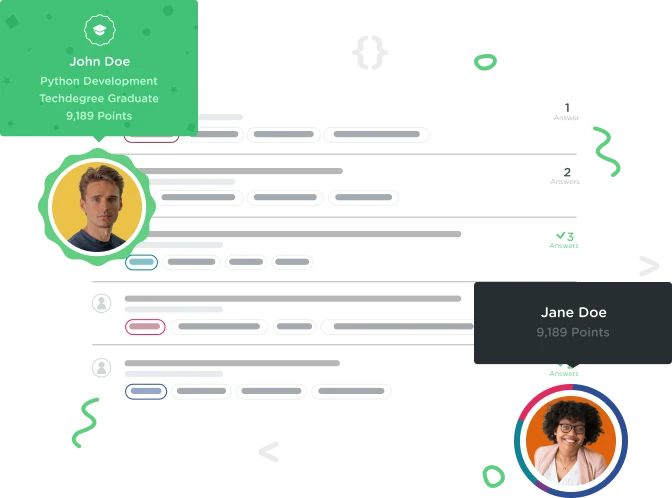

Andrew Stevens
11,083 PointsHow do I bind values from Visual Crossing?
Hi, As Dark Sky is no longer available I am using Visual Crossings API instead.
When I run the application in debugger I can see the data populates as expected, yet when I try to bind these values it throws a JSON Exception stating there are no values.
package com.shayledevops.stormy;
import androidx.annotation.NonNull;
import androidx.appcompat.app.AppCompatActivity;
import androidx.databinding.DataBindingUtil;
import android.app.AlertDialog;
import android.content.Context;
import android.net.ConnectivityManager;
import android.net.NetworkInfo;
import android.os.Bundle;
import android.text.method.LinkMovementMethod;
import android.util.Log;
import android.widget.TextView;
import android.widget.Toast;
import com.shayledevops.stormy.databinding.ActivityMainBinding;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.IOException;
import okhttp3.Call;
import okhttp3.Callback;
import okhttp3.OkHttpClient;
import okhttp3.Request;
import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather currentWeather;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
ActivityMainBinding binding = DataBindingUtil.setContentView(MainActivity.this,
R.layout.activity_main);
TextView visualCrossing = findViewById(R.id.visualCrossingAttribution);
visualCrossing.setMovementMethod(LinkMovementMethod.getInstance());
String apiKey = "YA4M8AJSQCCNKMUCR9SBP8EXD";
double latitude = 37.8267;
double longitude = 122.4233;
String forecastURL = "https://weather.visualcrossing.com/VisualCrossingWebServices/rest/services/timeline/"
+ latitude + "," + longitude
+ "?key=" + apiKey;
if(isNetworkAvailable()){
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastURL)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(@NonNull Call call, @NonNull IOException e) {
}
@Override
public void onResponse(@NonNull Call call, @NonNull Response response) throws IOException {
try {
String jsonDATA = response.body().string();
Log.v(TAG, jsonDATA);
if (response.isSuccessful()){
currentWeather = getCurrentDetails(jsonDATA);
CurrentWeather displayWeather = new CurrentWeather(
currentWeather.getLocationLabel(),
currentWeather.getIcon(),
currentWeather.getTime(),
currentWeather.getTemperature(),
currentWeather.getHumidity(),
currentWeather.getPrecipChance(),
currentWeather.getSummary(),
currentWeather.getTimezone()
);
binding.setWeather(displayWeather);
}
else {
alertUserAboutError();
}
} catch (IOException e) {
Log.e(TAG, "IO Exception Caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "JSON Exception caught: ", e);
}
}
});
}
Log.d(TAG, "Main UI code is running, hooray!");
}
private CurrentWeather getCurrentDetails(String jsonDATA) throws JSONException {
JSONObject forecast = new JSONObject(jsonDATA);
String timezone = forecast.getString("timezone");
Log.i(TAG,"From JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currentConditions");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("datetimeEpoch"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setLocationLabel("Alcatraz Island, CA");
// currentWeather.setPrecipChance(currently.getDouble("precipprob"));
currentWeather.setSummary(currently.getString("description"));
currentWeather.setTemperature(currently.getDouble("temp"));
currentWeather.setTimezone(timezone);
Log.d(TAG, currentWeather.getFormatedTime());
return currentWeather;
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager) getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
else {
Toast.makeText(this, R.string.network_unavailable_message,
Toast.LENGTH_LONG).show();
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogueFragment dialogue = new AlertDialogueFragment();
dialogue.show(getSupportFragmentManager(), "error_dialog");
}
}
Any ideas as to where I'm going wrong here?
bjoern schaefer
30 Pointsbjoern schaefer
30 PointsHi - i am actually working at Visual Crossing - this looks like you have some naming mismatch - we have a document listing the 'translations' of the fields : https://www.visualcrossing.com/resources/blog/how-to-replace-the-dark-sky-api-using-the-visual-crossing-timeline-weather-api/
that should resolve your issue
Cheers - Bjoern