Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial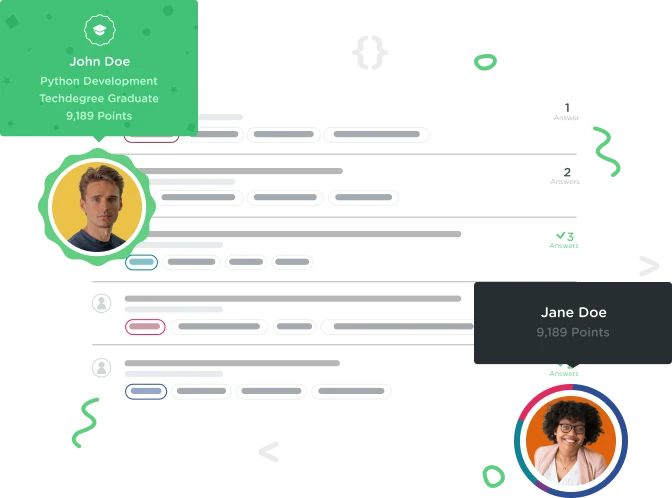
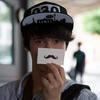
charlesmonaghan
742 PointsHow do I check for upper-case and lower-case?
I believe I got most of this right.
But now I can't figure out how to check for both lower-case and upper-case.
Could you please tell me the theory of how to do it without telling me the direct answer :)
def disemvowel(word):
what_to_remove = ["a", "e", "i", "o", "u"]
try:
word = word.remove(what_to_remove)
except ValueError:
pass
return word
2 Answers
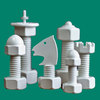
Steven Parker
231,269 PointsYou can compare both the lower-case and upper-case versions of a character separately as Jennifer suggested. Or if you are performing a comparison with a single letter, you could convert the case of that letter using the Python upper()
or lower()
functions and then just do a single comparison.
Another hint: it appears you're trying to use a "remove" method on the string "word", but strings do not have a "remove" method. Python strings are actually immutable (cannot be changed). Perhaps you were thinking of converting the string to a list first?
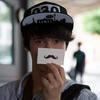
charlesmonaghan
742 PointsThanks for the second hint. I'm trying to get it working but for some reason .split() won't get the job done. How would I turn it into a list?
def disemvowel(word):
word = word.split()
what_to_remove = ["a", "e", "i", "o", "u", "A", "E", "I", "O", "U"]
try:
word = word.remove(what_to_remove)
except ValueError:
pass
return word
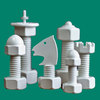
Steven Parker
231,269 PointsThe "split" is probably working just fine. But you can't give "remove" a whole list of things to remove (unless the other list as a sublist that matches it exactly). You might need a loop to remove one at a time.
And don't forget that both the argument and return values are strings, so if you convert to a list, you'll need to convert back before you return it.
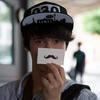
charlesmonaghan
742 PointsCould you give me an example of a loop that'll work? But don't give me a loop that matches the challenge.
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherThis is not an answer, but just a hint. Right now you have a list containing all the lower case vowels. Why not add the upper case vowels to that list as well?