Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial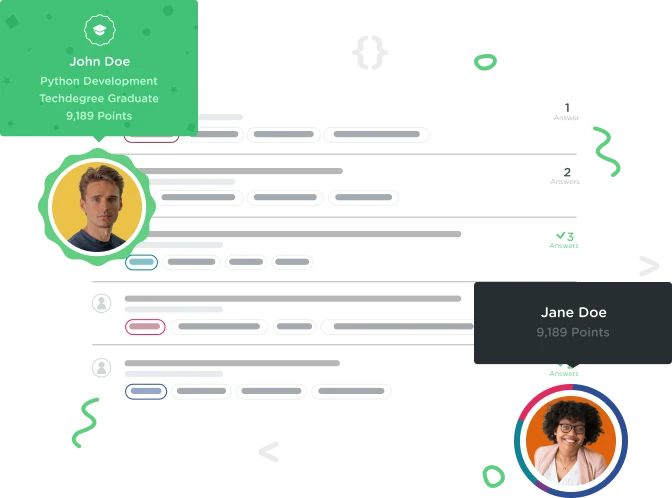

Haitao Huang
2,834 PointsHow do I check if a variable is an integer or not?
This challenge is similar to an earlier one. Remember, though, I want you to practice! You'll probably want to use try and except on this one. You might have to not use the else block, though. Write a function named squared that takes a single argument. If the argument can be converted into an integer, convert it and return the square of the number (num ** 2 or num * num). If the argument cannot be turned into an integer (maybe it's a string of non-numbers?), return the argument multiplied by its length. Look in the file for examples.
# EXAMPLES
# squared(5) would return 25
# squared("2") would return 4
# squared("tim") would return "timtimtim"
def squared(argument):
try:
if int(argument) == argument:
return int(argument) ** 2
except ValueError:
return argument * len(argument)
squared("cake")

Haitao Huang
2,834 PointsThank you! Chris. I just added my attempt to the board.
1 Answer
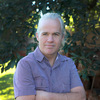
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsYou almost have it, only squared("2") would return 4 will not work and that is quite easliy sorted by removing the line if int(argument) == argument: as this only lets original integers pass.
def squared(argument):
try:
return int(argument) ** 2
except ValueError:
return argument * len(argument)

Haitao Huang
2,834 PointsProblem solved! Thank you so much! :)
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsChristopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsGood day,
You have not put a question, only pasted the challege question that is available anyway.
The community can help but it is more helpful for you to show what you have attempted and the community and assist you by pointing out where you are going wrong and point you in the right direction so that you understand the problem and solution.