Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial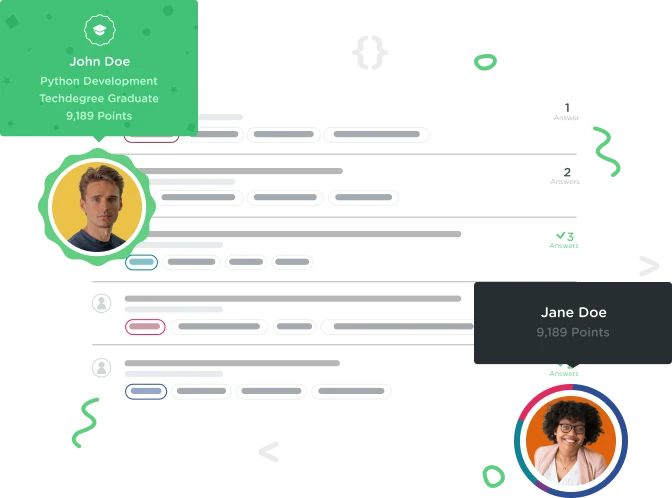
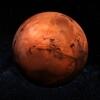
Duane Smith
1,919 PointsHow do I compare instances from a given class?
I'm trying to create methods that return a true/false Boolean value that will compare two different instances in terms of their length. I feel like I'm either using the wrong variable or I'm not using the right method to compare instances, but either way I'm fairly lost as to where I should go.
Any help will be appreciated!
Thanks in advance!
class Song:
def __init__(self, artist, title, length):
self.artist = artist
self.title = title
self.length = length
def __eq__(self, new):
if not isinstance(new, Song):
return NotImplemented
return self.title == new.title and self.artist == new.artist
def __lt__(self, new):
if not isinstance(new, Song):
return NotImplemented
return self.title > new.title and self.artist > new.artist
def __gt__(self, new):
if not isinstance(new, Song):
return NotImplemented
return self.title < new.title and self.artist < new.artist
def __le__(self, new):
if not isinstance(new, Song):
return NotImplemented
return self.title <= new.title and self.artist <= new.artist
def __ge__(self, new):
if not isinstance(new, Song):
return NotImplemented
return new.title >= self.title and new.artist >= self.artist
1 Answer
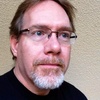
Chris Freeman
Treehouse Moderator 68,441 PointsThe question is precisely how do you wish to compare objects by length
? Standard comparison operators map directly to the dunder methods already defined. If you now wish to use the standard comparison operators the above methods would need to be redefined to use self.length
instead of name and title.
def __gt__(self, new):
if not isinstance(new, Song):
return NotImplemented
return self.length < new.length
If you do not wish to use the standard comparison operators, there are a few options:
use direct comparisons of length:
song1.length > song2.length
add a
__len__
method that returns theself.length
. Then, you could comparelen(song1) > len(song2)
you could create a new "regular" method, such as
gt_len
, using the same form as above as the other dunder methods. The new method could be called asinstance1.gt_len(instance2)
a really cheeky alternative: override the modulo operator %. which calls the
__mod__
dunder method. This would be very non-standard and not recommended.
def __mod__(self, new):
"""returns True if two instance lengths equal
Usage:
if song1 % song2:
print(f"{song1} and {song2} have equal lengths")
"""
if not isinstance(new, Song):
return NotImplemented
return self.length == new.length
post back if you wish more help. Good luck!!
Duane Smith
1,919 PointsDuane Smith
1,919 PointsWow.. Chris Freeman Thank You.
These examples and explanations are amazing!! On my own, I was trying to go more something more like option 1 or possibly option 3. I felt the code would just be more self-explanatory or readable to any programmer that would look at it down the road. I'm trying to train myself as if I'm writing programs for a team that may need to get reviewed or revisited.
This is awesome and exactly what I was looking for in getting help!
I truly appreciate it.
Best,
Duane Smith