Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial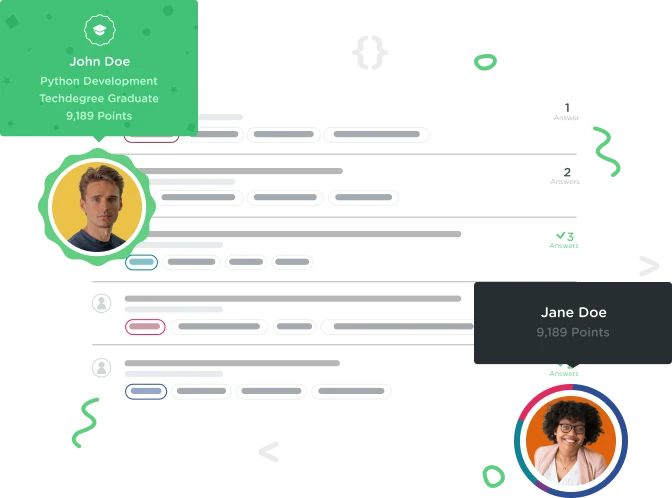
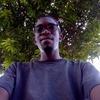
Elias Svoba
14,347 PointsHow do i complete the loop for the Boggle game board?
char[][] boggle = { {'C', 'A', 'T'}, {'D', 'R', 'I'}, {'L', 'O', 'G'} };
System.out.printf("-------------%n"); for (int __ = 0; < boggle.length; __ ++) { for (int __ = 0; < boggle[].length; __ ++) { System.out.printf("| %s ", boggle[][]); } System.out.printf("|%n-------------%n"); }
The __ needs to be filled with a standard variable name. I tried using i & j as illustrated int the video but it is not passing.
2 Answers

Rich Zimmerman
24,063 PointsTry to think about what each for loop is looping over, and also think about how you reference a value with in a multi-dimensional array.
The 2nd for loop is nested inside of the first, so the first loop will iterate over each array, while the 2nd for loop will iterate over each item in an array before the top level loop will go to the next index.
When you reference an item in a multi-dimensional array you need the index of the array and the index of the value you're referencing.
char[][] boggle = {
{'C', 'A', 'T'},
{'D', 'R', 'I'},
{'L', 'O', 'G'}
};
// ex.
boggle[0][1] // is "A"
So your for loop would look like this.
System.out.printf("-------------%n");
// this loops over each index in the top level of the multi-dimensional array (or each array within the array)
for (int i = 0; i < boggle.length; i++) {
// this loops over each index in the array that the top level for loop is currently at in it's for loop
for (int j = 0; j < boggle[i].length; j++) {
System.out.printf("| %s ", boggle[i][j]);
}
System.out.printf("|%n-------------%n");
}
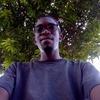
Elias Svoba
14,347 PointsThanks a lot!
Elias Svoba
14,347 PointsElias Svoba
14,347 PointsIn this line for me it was (for (int j = 0; j < boggle[j].length; j++) {) instead of (for (int j = 0; j < boggle[i].length; j++) {) but i managed to follow your explanation and i now fully understand the concept.
r j weiner
2,741 Pointsr j weiner
2,741 Pointsniiiicccceeee