Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial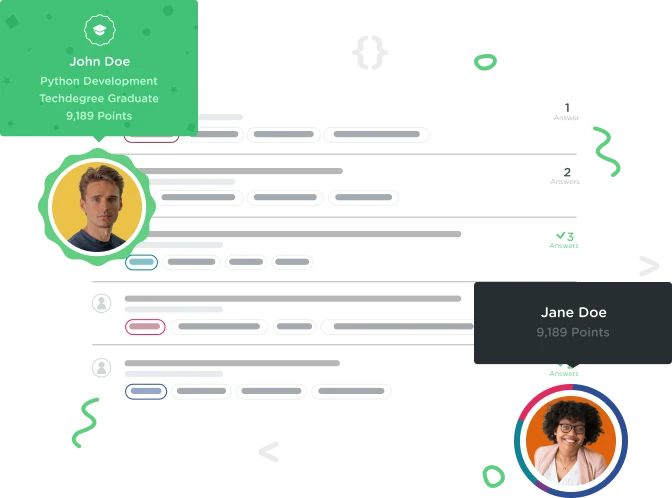
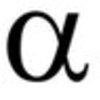
peter peter
9,151 Pointshow do i connect an android app to MongoDB on a remote server? how do i parse the JSON response?
I need pub/sub functionality; thereby updating my android app when new information becomes available. my server is privately hosted. the server stack entails express.js and mongoDB.
2 Answers

Chris Wolchesky
12,025 PointsYou'd have to create some sort of middle-ware API to interact with the database on behalf of your Android app. Express is great at this, alternatively I also like Restify (http://restify.com/) for creating a quick Node.js REST API. Within Android, you can interact with this API a couple of different ways. The easiest way is to use a library such as Retrofit (http://square.github.io/retrofit/) to essentially use your API as a Java Interface. Alternatively, you can use an AsyncTask (http://developer.android.com/reference/android/os/AsyncTask.html) to create a request to the API endpoint URL manually and load the resulting InputStream object into a JSONObject to retrieve the data. Here's a quick example of that at work using the Cat Facts API:
private class CatFactsActivity extends AsyncTask<Object, Void, JSONObject> {
@Override
protected JSONObject doInBackground(Object... params) {
JSONObject jsonResponse = null;
int responseCode;
try {
URL requestUrl = new URL("http://catfacts-api.appspot.com/api/facts");
HttpURLConnection connection = (HttpURLConnection) requestUrl.openConnection();
connection.setRequestProperty("Accept-Encoding", "identity");
connection.connect();
responseCode = connection.getResponseCode();
if (responseCode != HttpURLConnection.HTTP_OK) {
Log.e(TAG, "Status Code: " + responseCode);
} else {
InputStream stream = connection.getInputStream();
Reader reader = new InputStreamReader(stream);
int contentLength = connection.getContentLength();
if (contentLength > 0) {
char[] buffer = new char[contentLength ];
reader.read(buffer);
Log.d(TAG, buffer);
jsonResponse = new JSONObject(String.valueOf(buffer));
} else {
String jsonData = isToString(stream);
jsonResponse = new JSONObject(jsonData);
}
Log.d(TAG, jsonResponse.toString(2));
connection.disconnect();
}
}
catch (Exception e) {
Log.e(TAG, "Exception: ", e);
}
return jsonResponse;
}
protected void onPostExecute(JSONObject data) {
mFactJSON = data;
}
protected String isToString(InputStream is) {
ByteArrayOutputStream buffer = new ByteArrayOutputStream();
int nRead;
byte[] data = new byte[65536];
try {
while ((nRead = is.read(data, 0, data.length)) != -1) {
buffer.write(data, 0, nRead);
}
buffer.flush();
} catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
return buffer.toString();
}
}
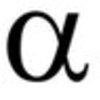
peter peter
9,151 Pointsbrilliant. thanks, Chris. much appreciated.