Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial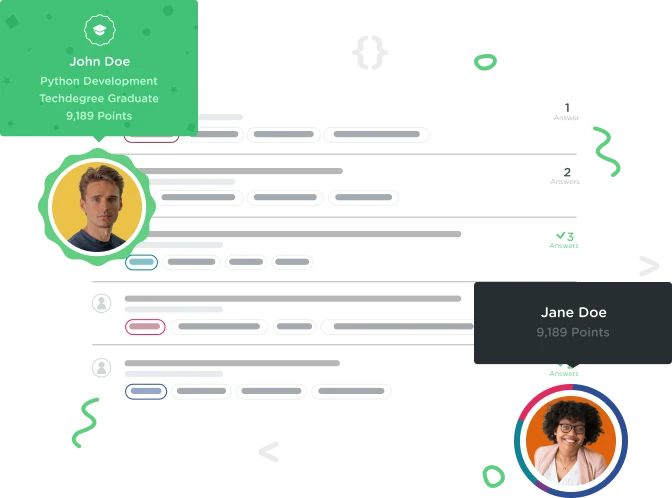
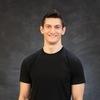
Joseph Butterfield
Full Stack JavaScript Techdegree Graduate 16,430 PointsHow do I console.log() inside of a Class?
So when Guil console logs "this.removePlayer" to show in the console the function passed from "App" to "Player", he does so with the Player Component written as a variable
const Player = (props) => {
console.log(props.removePlayer)
return(
JSX the player stuff
)
}
Though, not in the strict recommendation of Classes vs. Variables use because Player does not currently contain state, I still wrote my component as a Class like so:
class Player extends React.Component {
console.log(this.props.removePlayer)
render() {
return (
JSX the player stuff
)
}
}
I know the syntax changes from props.WHATEVER to this.props.WHATEVER, but when I try to console log from inside the class, I get the following error:
Uncaught SyntaxError: http://port-80-ez61genq7v.treehouse-app.com/app.js: Unexpected token (9:9)
7 |
8 | class Player extends React.Component {
9 | console.log(this.props.removePlayer);
I loaded the code into babeljs.io and I got a transpiler error. So how do I console.log inside of a Class like you simply do inside of a variable? It seems as if both components should simply require different arguments to the console.log to work..
1 Answer

Torben Korb
Front End Web Development Techdegree Graduate 91,433 PointsHi Joseph, either you call the console.log statement inside the Class constructor like:
class Player extends React.Component {
constructor(props) {
super(props);
console.log(this.props.removePlayer)
}
render() {
return (
// JSX the player stuff
);
}
}
which will call the log before the component is mounted or you call the statement inside the render method like:
class Player extends React.Component {
render() {
console.log(this.props.removePlayer)
return (
// JSX the player stuff
);
}
}
This will call the log anytime the component renders. You can't write code plain inside the block of your Class at the moment. Only inside methods of the Class or the constructor.
See more explanation to JavaScript Classes here: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Classes
Hope this helps you! Happy coding!