Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial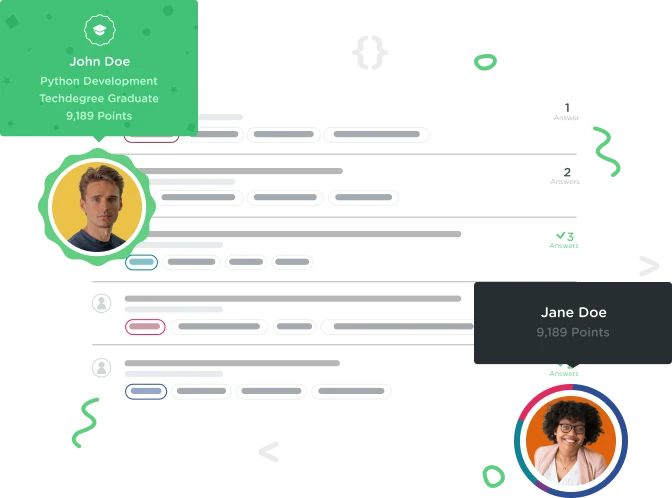

Zachary Maldonado
5,895 PointsHow do I convert a string argument into a keyword?
I believe the rest of code is correct and the part that's messing me up is that on timedelta() function I pass in a string instead of keyword for the first argument(cause thats what the time_machine function takes). Its probably something really simple that I have to do, but I just cant figure it out. Please help
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
def time_machine(integer, increment):
new_time = starter + datetime.timedelta(increment = integer)
return new_time
time_machine(5, "hours")
2 Answers
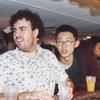
Brent Liang
Courses Plus Student 2,944 PointsHey Zach,
I was having the exact same problem. What I did was having a nested if statement with multiple elifs to cover each scenario - apparently string argument can't be used for keyword in timedelta.
I've attached my code below, hope it offers some inspiration!
import datetime
starter = datetime.datetime(2015, 10, 21, 16, 29)
# Remember, you can't set "years" on a timedelta!
# Consider a year to be 365 days.
def time_machine (num, type1):
if type1 in ["minutes", "hours", "days"]:
if type1 == "minutes":
endtime = starter + datetime.timedelta (minutes = num)
return endtime
elif type1 == "hours":
endtime = starter + datetime.timedelta (hours = num)
return endtime
elif type1 == "days":
endtime = starter + datetime.timedelta (days = num)
return endtime
elif type1 == "years":
endtime = starter + datetime.timedelta (days = 365*num)
return endtime
else:
print("Invalid!")
## Example
# time_machine(5, "minutes") => datetime(2015, 10, 21, 16, 34)
```python

Ryan S
27,276 PointsHi Zachary,
You don't need to call the function at the end. The challenge does this for you and will pass in whatever values it wants in order to test your code.
The other thing is that you can't substitute keyword names with variables. For example, in datetime.timedelta()
, there are the keywords "hours", "minutes", "days", etc... Unfortunately you cannot set increment = "hours"
then increment = integer
. Although it seems to make sense, it doesn't actually work.
However, there is a way to do this by creating a dictionary of 'increment' and 'integer' and then unpacking it in timedelta. Or you can simply use if
statements to handle each case.
Good luck
Zachary Maldonado
5,895 PointsZachary Maldonado
5,895 PointsThanks a lot Brent. I was having a tough time with this one. The string to keyword problem is something I'm gonna have to look more into. I couldn't seem to get it using dictionaries like Ryan had suggested. Happy coding!