Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial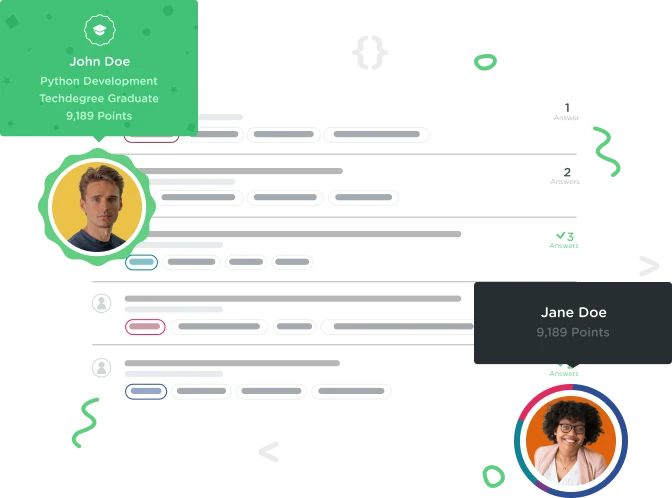

Rasheed Ajala
Courses Plus Student 2,003 Pointshow do i convert an argument in my function to float. This is python.
Let's make sure we're always working with floats. Convert your arguments to floats before you add them together. You can do this with the float() function.
The first question says to create a function add that takes two arguments and returns their sum. The second question is above.
This is what I did and I think should be correct: def add(x,y): return(float(x +y)
It worked on my python shell on my computer but i keep getting a wrong feedback in the quiz.
28 Answers
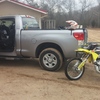
Ryan Ruscett
23,309 PointsHola,
So the question says
create a function add the two arguments and returns their sum
def add(x , y):
return x + y
At this point we assume we are just adding numbers together.
Let's make sure we're always working with floats. Convert your arguments to floats before you add them together. You can do this with the float() function.
Ok let's make sure no matter what comes in it's converted to a float
def add(x , y):
a = float(x)
b = float(y)
return a + b
Ok now I converted each value to a float. It could be any whole number or float passed in, and no matter what it is, I am going to turn it into a float.
BUT WAIT? What happens if I pass in a string? That can't be converted to a float. When a string like "hello" is converted into a float, it fails. It gives you a ValueError. Meaning the value can't be converted to float. can't convert a text string into a float. So we catch that and return nothing. Otherwise we were able to convert them both successfully and return true.
This might be the 3/3 in the challenge I think.
def add(x, y)
try:
a = float(x)
b = float(y)
except ValueError:
return None
else:
return True
Let me know if this makes sense or not. If not I can attempt to approach it another way.
Thanks!

Felipe Marconatto Ochman
4,494 Pointshi, this work for me!
def add(a,b): return float(a) + float(b)

william Murray
1,337 PointsThanks! This worked.

sebastian tramper
7,210 PointsThanks!

Vedran Linić
Courses Plus Student 18,802 PointsFore final challange this is the code that i used
def add(num1, num2): try: return float(num1) + float(num2) except ValueError: return None else: print(num1 + num2)

paul chon
1,605 Pointsassign the process of adding the two floats into a variable.
def add(x, y):
try:
a = float(x)
b = float(y)
total = a + b
except ValueError:
return None
else:
return total
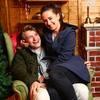
Patric Daniel Pförtner
1,542 PointsHi Rasheed,
You are very close to the answer, here is how I did it:
def add(num1, num2):
a = float(num1)
b = float(num2)
return(a + b)
Why are you learning Python? Maybe we have the same aims! I am learning it to bring my Start UP www.wolf-gate.com to the next level. Thank´s to Kenneth Love it´s easily possible :)

Thomas Souza
7,943 Pointsdef add(num1, num2): a = float(num1) b = float(num2) return (a+b)
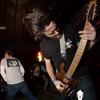
Patrick Chumley
6,009 PointsYour problem is having to do with your syntax. Your return needs two sets of parentheses like this:
def add(x, y): return(float(x + y))
That should fix the issue you are encountering!

Rasheed Ajala
Courses Plus Student 2,003 PointsI added the parenthesis in my original code, that was an omission. Thanks for catching that

Rasheed Ajala
Courses Plus Student 2,003 Pointscan I see your code?

Petrius Balbinot
1,805 PointsNone of the codes posted above work, correct?
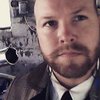
Daniel Dugan
16,904 PointsYou will want to modify the else statement.
else:
print(a + b)

Aaron Mckenzie-Hunte
10,741 PointsHi,
If you are still stuck, try this:
def add (num1, num2): try: num1 = float(num1) num2 = float(num2) except ValueError: return None else: return (float(num1 + num2))
This is the solution that allowed me to pass Q 3/3

Peter Rzepka
4,118 Pointsdef add(a, b): try: a = float(a) b = float(b) except ValueError: return None else: return(float(a + b))

Kong Cheong
633 PointsHi, I followed the steps but unable to get it to work. I completed 1/3 and 2/3 perfectly then 3/3 is returning error every tiime I submit. Please help, I have no idea what is wrong.

Kong Cheong
633 PointsHi Rasheed,
Thank you for reaching out. I posted a discussion and they helped me figured it out. So I am good to go. I believe my code were correct and there is some spacing/indent issues I have.
I appreciate it a lot for replying! Happy Holidays!
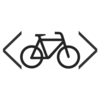
Kory Hoopes
3,099 PointsWhat am I doing wrong here?
def add(x, y)
try:
a = float(x)
b = float(y)
except ValueError:
return None
else:
return True
a = float(x)
b = float(y)
return a + b

Zeljko Porobija
11,491 Pointselse should only have the return statement. No other statements are needed at all.

jasonhass
2,417 PointsDo not know if you are still monitoring this thread, however, you are missing a colon after...
def add(x, y):
...and then clean up your else statement (indents where appropriate of course)...
else: return x + y

Ivan K
1,928 Pointshelle everybody!
do you know, why my code doesn't work?:
def add(one, two):
try:
o = float(one)
t = float(two)
except ValueError:
return None
else:
return (o+t)

youssef moustahib
2,303 PointsHow is a float a letter?
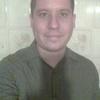
Cristobal Ortiz-Ortiz
1,000 Pointshad try different variant without luck what i am missing???
def add(hola,hello):
try
a = float(hola)
b = float(hello)
except ValueError:
return None
else:
return(float(a + b))
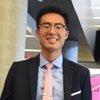
Paul Je
4,435 PointsIs there a complete answer? Haha I tried mine using some of the tips on here and still can't figure it out.. my code looks like this:
def add(x, y): try: a = float(x) b = float(y) except ValueError: return None
else: return True return(a + b)

Jarrod Gillis
16,760 PointsBASIC CONFIGURATION:
def add(points, rebounds): points = float(points) rebounds = float(rebounds)
return(points + rebounds)
WITH TRY AND EXCEPT:
def add(points, rebounds):
try:
points = float(points)
rebounds = float(rebounds)
except ValueError:
return None
else:
return(points + rebounds)

Bo Winters
Full Stack JavaScript Techdegree Student 1,481 Pointsdef add(a, b): try: a = float(a) b = float(b) except ValueError: return None else: return(float(a + b))
After trying everything else, this one finally worked for me!

Aron Katz
700 PointsI am getting no where fast on this last challenge too, it's been wasting a lot of my time and makes no sense, I have just about had enough.
def add(num1, num2):
try:
a = float(num1)
b = float(num2)
except ValueError:
return None
else:
return (float(a + b))
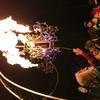
ANDY VAN DEN BOS
2,330 Pointsthe answer: def add(num1, num2): try: a = float(num1) b = float(num2) except ValueError: return None else: return a + b
Now keep in mind "try, except, and else" must all be in line with each other as in- properly indented.
Hope someone finds this sooner then It took me!

reza rahnama
Python Web Development Techdegree Student 1,118 Pointsdef add (exe1, exe2): try: a = float (exe1) b = float (exe2) total = a + b
except ValueError:
return None
else:
return total
I have tried various combinations and not able to get this to work, Can some one advise whats wrong with the above syntax as logically I can't figure it out why it wouldn't work
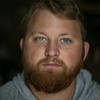
kevin O'Brien
508 PointsMake sure all of the indentations are correct! I input all of these codes and the only thing incorrect was improper indentation. So if you're stuck and you know your syntax is correct, make sure the indents are proper! Thanks!

piper piperian
8,958 PointsYes, for me the prob was the indentation! So even with a correct code, it will throw an error if you are not watchful of the spacings.

Rehan Hussain
Courses Plus Student 2,199 PointsGusy - Why isn't this code running this is so frustrating! They need to have a live chat or something, such a waste of time!
def add(x, y):
try:
a = float(x)
b = float(y)
except ValueError:
return None
else:
return x + y

bviengineer
16,484 PointsThe following worked for me:
def add(num1, num2): try: num1F = float(num1) num2F = float(num2) except ValueError: return None else: total = num1F + num2F return total

B W
2,523 Pointsdef add(dance, play): try: a = float(dance) b = float(play) except ValueError: return None else: total = a + b return total

Jason Turla
2,575 PointsThis worked for me. What I seem to have done differently than what others have shown is making 'total' a variable and return the variable. In the first part total = a + b then it needs to be changed since they were changed to a float.
def add(a,b): try: x = float(a) y = float(b) total = x + y return total except ValueError: return None else: return total
Rasheed Ajala
Courses Plus Student 2,003 PointsRasheed Ajala
Courses Plus Student 2,003 PointsThanks it worked. But do you know why mine didn't? keeping in my mind that I added all parentheses.
Ryan Ruscett
23,309 PointsRyan Ruscett
23,309 PointsYup I do.
First thing is first.
If you write (float(x + y) <---- you forgot to close a parentheses A: return (float(x + y)) <--- need TWO
This is how what you are saying is being interpreted.
return(float(x + y)
Say I am Python....
first I use order of operation in a sense. I will do the first bit of code in () Which is x = y. Ok cool I did that. Now I move outward to the second set (float( ) Which is float. So now I convert the value of x + y to a float. Then I return a float.
BUT WAIT!!!
I don't want to return a float. i want to make sure the two values I am adding are floats and if they are not floats, than I need to fail.
Does that help?
Rasheed Ajala
Courses Plus Student 2,003 PointsRasheed Ajala
Courses Plus Student 2,003 PointsOhh right. Yeah that solves my problem. Didn't understand the question enough. lol, thanks man.
Ryan Ruscett
23,309 PointsRyan Ruscett
23,309 PointsAwesome! Good Luck pythonista!
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsThis only worked for me when I kept a as a and b as b instead of turning them into x and y...
a = float(a) b = float(b)
Levis Vazquez
961 PointsLevis Vazquez
961 PointsThank you !
Zeljko Porobija
11,491 PointsZeljko Porobija
11,491 PointsIs else: intended properly? It seems to me it's not, you added an extra space.
Megan Riley
5,150 PointsMegan Riley
5,150 PointsAny reason why this wouldn't work for step 3?
def add(x, y) try: a = float(x)
b = float(y) except ValueError: return None else: return True
Paul Je
4,435 PointsPaul Je
4,435 Pointsif you copy and paste this code, it doesn't work, is it because you need to add a return statement below the returned True statement? I tried that as well but maybe the order in which you place it either in the try block or else block may matter or have some different unique syntax attached to it..
Donal Lane
Courses Plus Student 3,140 PointsDonal Lane
Courses Plus Student 3,140 PointsI did this and its saying "Oops! Looks like Task 1 is no longer passing."
What am I doing wrong?