Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial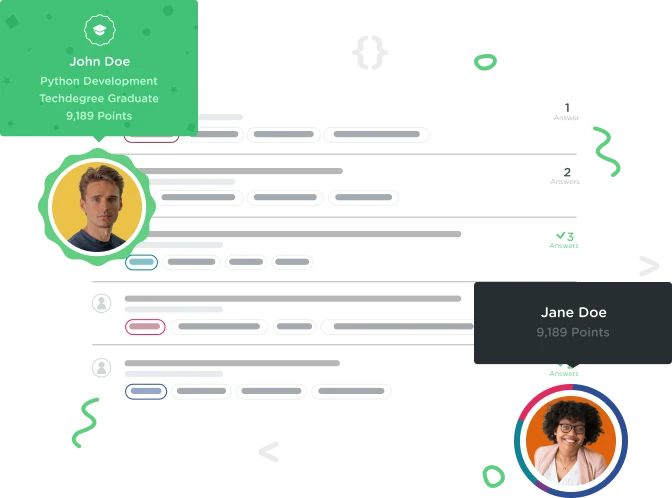
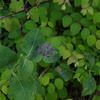
Fabian Pijpers
Courses Plus Student 41,372 PointsHow do I convert the Gpa value to string anybody?
How do I convert a numerical value to a string value in Javascript and then return it?
class Student {
constructor(gpa){
this.gpa = gpa;
}
stringGPA(gpa)
{
return gpa.toString;
}
}
const student = new Student(3.9);
2 Answers
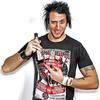
Andreas Nyström
8,887 PointsThe easiest way to create a string is to add an empty string to it.
stringGPA(){
// will return the gpa as a string.
return this.gpa + "";
}
Could also mention to make a number out of a string, (the string has to hold a number, but as a string variable), the easiest way is probably to divide it or multiply it by 1
let stringNum = "22"
// will console.log 22 as a number.
console.log(stringNum / 1)

Tim Knight
28,888 PointsHi Fabian,
You're on the right track with the use of toString()
, however remember it's a function and needs ()
at the end. Additionally, since you're passing in the GPA when you initialize the class there's really no reason to pass it in again, you can just reference the GPA value from the class (which is this
). Try modifying your class to look something like this.
class Student {
constructor(gpa){
this.gpa = gpa;
}
stringGPA() {
return this.gpa.toString();
}
}
const student = new Student(3.9);
console.log(student.stringGPA());
Now calling the stringGPA()
function on the instance of your class will return the value of the GPA as a string.
Tim Knight
28,888 PointsTim Knight
28,888 PointsThese certainly work Andreas but often just using
toString()
can be clearer for other developers looking at your code. To then convert to a number from a string you haveparseInt()
,number()
, andparseFloat()
which can all address versions of this feature without having to do equation tricks.Andreas Nyström
8,887 PointsAndreas Nyström
8,887 PointsTim Knight I've yet to see someone who prefers to use these methods over the one I mentioned. But I'm glad we're different. Let me try to explain why I prefer these methods so people get both versions.
toString() does exactly + "", but doesn't work on numbers directly. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/toString