Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial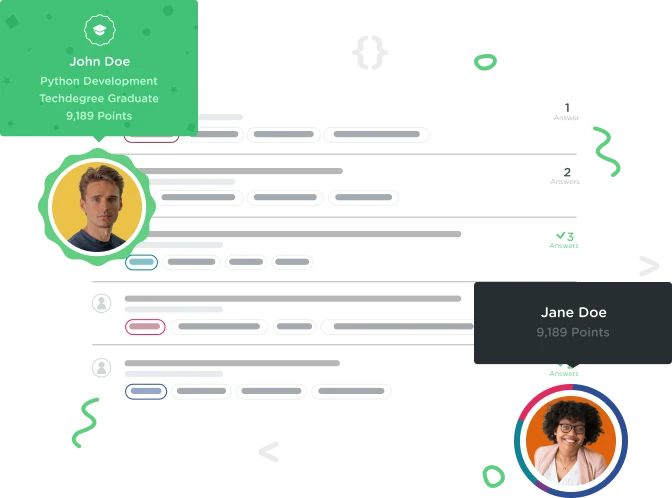
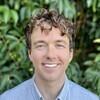
Asher Orr
Python Development Techdegree Graduate 9,410 PointsHow do I correctly reference a variable within a method (OOP)?
Hi everyone! In the "summary" method (at the bottom of the file quiz_wf.py), I'm trying to print out how many seconds it took to answer each question.
I'm working with 2 files:
File 1: questions.py
class Question:
answer = None
text = None
class Add(Question):
def __init__(self, num1, num2):
self.text = '{} + {}'.format(num1, num2)
self.answer = num1 + num2
class Multiply(Question):
def __init__(self, num1, num2):
self.text = '{} x {}'.format(num1, num2)
self.answer = num1 * num2
File 2: quiz_wf.py
import datetime
import random
from questions import Add, Multiply
class Quiz:
questions = []
answers = []
def __init__(self):
question_types = (Add, Multiply)
num_questions = int(input("How many questions would you like on the quiz? "))
starting_range = int(input("For all your questions, what's the lowest number you'd like to work with? "))
ending_range = int(input("For all your questions, what's the highest number you'd like to work with? "))
for _ in range(num_questions):
num1 = random.randint(starting_range, ending_range)
num2 = random.randint(starting_range, ending_range)
question = random.choice(question_types)(num1, num2)
self.questions.append(question)
def take_quiz(self):
self.start_time = datetime.datetime.now()
for question in self.questions:
self.answers.append(self.ask(question))
else:
self.end_time = datetime.datetime.now()
return self.summary()
def ask(self, question):
correct = False
question_start = datetime.datetime.now()
answer = input(question.text + ' = ')
if answer == str(question.answer):
correct = True
question_end = datetime.datetime.now()
return correct, question_end - question_start
def total_correct(self):
total = 0
for answer in self.answers:
if answer[0]:
total += 1
return total
def summary(self):
print(f"You got {self.total_correct()} out of {len(self.questions)} right.")
print(f"It took you {(self.end_time - self.start_time).seconds} seconds for the whole quiz.")
print("For each question, this is how many seconds you took: ")
my_quiz = Quiz()
my_quiz.take_quiz()
I am new to OOP, and I am struggling with this task.
In my "summary" method, I tried this code:
def summary(self):
print(f"You got {self.total_correct()} out of {len(self.questions)} right.")
print(f"It took you {(self.end_time - self.start_time).seconds} seconds for the whole quiz.")
print("For each question, this is how many seconds you took: ")
for question in self.questions:
print(f'Question #{self.questions.index(question)+1}: {self.question_end - self.question_start}.seconds')
I get this AttributeError, though:
AttributeError: 'Quiz' object has no attribute 'question_end'. Did you mean: 'questions'?
Can anyone help me with properly referencing the question_start and question_end variables in the "ask" method?
Thank you for reading!
1 Answer
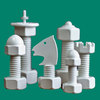
Steven Parker
231,275 PointsAs the message says, there's no "question_end" in the "questions" list. But the "ask" method returns a tuple containing the result status and a timedelta for the elapsed time, and that tuple gets appended to the "answers" list in "take_quiz".
So you can access the time from the "seconds" attribute of the timedelta. It might also be easier to have the loop step directly through the index values (instead of searching for them), for example:
for i in range(len(self.questions)):
print(f'Question #{i+1}: {self.answers[i][1].seconds}')
Asher Orr
Python Development Techdegree Graduate 9,410 PointsAsher Orr
Python Development Techdegree Graduate 9,410 PointsThis makes so much sense! Thank you, Steven. I didn't perceive the last line (return correct, question_end - question_start) in the "ask" method as a tuple.
I see now that you can loop through the range of self.questions, then use the answers list to display the time required for each. Thanks again!