Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial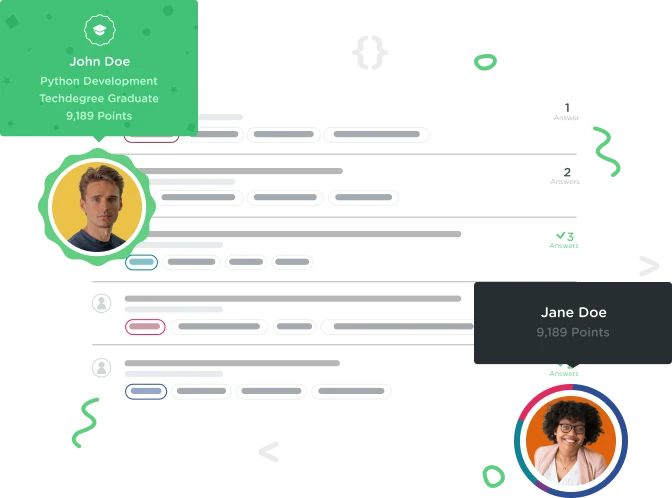

iwgpnjvycw
8,577 PointsHow do I correctly use an imported function to update state in a React component?
Hi all, I've been really struggling with how to properly use an imported function in a separate React component. The function I'm importing uses Axios to fetch a random cat image from http://aws.random.cat/meow.
I know catApi.js is correctly fetching the data, but I haven't been able to figure out how to use fetchRandomCat()
inside componentDidMount()
to update State. The error I'm getting right now is Cannot read property 'then' of undefined
.
What I need is to be able to get a new random cat with the click of a button. Any help would be greatly appreciated.
catApi.js
import axios from 'axios';
export function fetchRandomCat() {
axios.get('http://aws.random.cat/meow').then(function (response) {
console.log(response);
})
.catch(function(error) {
console.log('Error fetching cat', error);
})
}
CatLoader.js
import React from 'react';
import { fetchRandomCat } from './catApi';
export class CatLoader extends React.Component {
constructor(props) {
super(props);
this.state = {
cat: ''
};
}
componentDidMount() {
fetchRandomCat().then(function(response) {
this.setState({ cat: response.data.file });
});
}
render() {
return <div>{/* TODO - load a cat image from the cat api */}</div>;
}
}
1 Answer

Wiktor Bednarz
18,647 PointsI think that it's because you aren't returning a promise from your fetchRandomCat() on the componentDidMount() event:
Try with:
export function fetchRandomCat() {
return axios.get('http://aws.random.cat/meow').then(function (response) {
console.log(response);
})
.catch(function(error) {
console.log('Error fetching cat', error);
})
}