Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial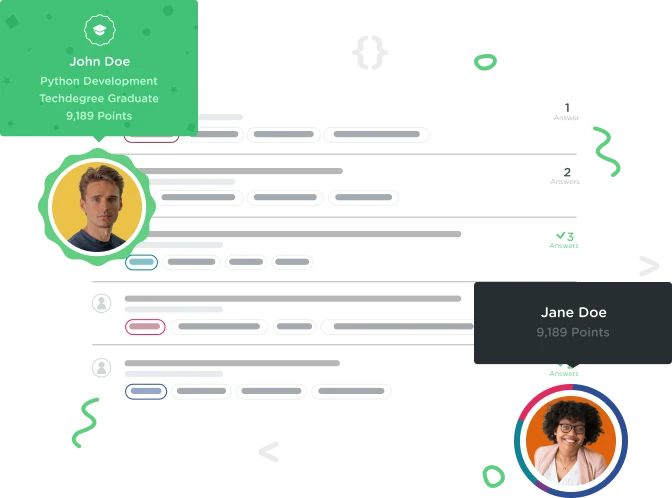

KORAXE IUHMAGEON
Courses Plus Student 8,486 PointsHow do I create a method on the object using the code in the function at the top
Help I need a badge before I can sleep
function printFullName() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
var contact = {
}
contact.fullName = function(firstName, lastName) {
return printFullName();
}
2 Answers
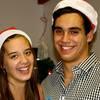
Cody Te Awa
8,820 PointsHi there Koraxe, to add a method to an object you can simply do it the same as you would an attribute. So if you were to add an attribute you might go about it like this:
var contact = {
//a first name attribute
firstName: Cody
//a last name attribute
lastName: Te Awa
}
Now to add a method to this object you can do it like this:
var contact = {
//a first name attribute
firstName: Cody
//a last name attribute
lastName: Te Awa
//A method that returns my full name
fullName: function() {
console.log(this.firstName + ' ' + this.lastName)
}
}
In this example though there are no attributes required AND the function is provided so you can simply do:
var contact = {
fullName: printFullName
}

KORAXE IUHMAGEON
Courses Plus Student 8,486 Pointsthank you but the next question asks me to make fullName an anonymous function. I do not know what an anonymous function is yet.
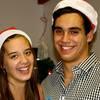
Cody Te Awa
8,820 PointsAn anonymous function is just an unnamed function. So using my previous example it might be confusing. Instead they would like you to have something like this:
var contact = {
fullName: function printFullName() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
}
However that example is a named function and is unnecessary. There is no need for us to call it printFullName we can now just delete that part and voila!
var contact = {
fullName: function() {
var firstName = "Andrew";
var lastName = "Chalkley";
console.log(firstName + " " + lastName);
}
}
Notice in the latter that I have removed "printFullName" :) Happy Coding!