Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial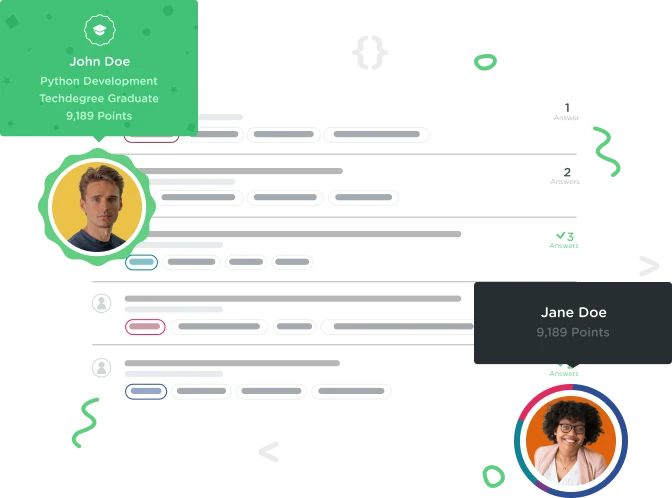

shazza69
Courses Plus Student 691 PointsHow do I create a React Consumer within a component file itself?
I'm trying to call withContext directly from a component file instead of from App.js. This is my Context.js file:
import React, { Component } from 'react'
import Data from './Data'
const Context = React.createContext()
export class Provider extends Component {
constructor() {
super()
this.data = new Data()
}
render() {
const value = { data: this.data }
return (
<Context.Provider value={value}>{this.props.children}</Context.Provider>
)
}
signIn = async () => {}
signOut = () => {}
}
export const Consumer = Context.Consumer
/**
* A higher-order component that wraps the provided component in a Context Consumer component.
* @param {class} Component - A React component.
* @returns {function} A higher-order component.
*/
export default function withContext(Component) {
return function ContextComponent(props) {
return (
<Context.Consumer>
{context => <Component {...props} context={context} />}
</Context.Consumer>
)
}
}
And this is my UserSignUp.js page:
import React, { Component, Fragment } from 'react'
import Link from 'next/link'
import withContext from '../Context'
class UserSignUp extends Component {
// yada yada yada
submit = () => {
const { context } = this.props
const { name, email, password } = this.state
const user = { name, email, password }
context.data
.createUser(user)
.then(errors => {
if (errors.length) {
this.setState({ errors })
} else {
console.log(`${email} is successfully signed up and authenticated!`)
}
})
.catch(err => {
console.log(err)
this.props.history.push('/error')
})
}
}
export default withContext(UserSignUp)
It works fine until I submit, and then it crashes saying "Can't read property of undefined", where undefined refers to the context.data line. I'm not sure why it's doing this. Could someone please help?
1 Answer
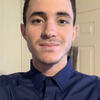
Oliver Duran
Full Stack JavaScript Techdegree Graduate 22,828 PointsHad the same problem and it was just a typo in my Context.js file I typed "vaule" instead of value on the "Context.provider" component.