Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial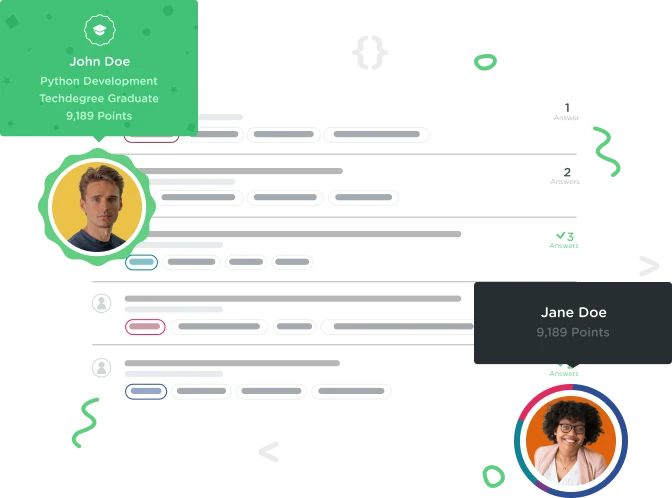
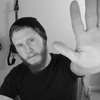
Jeremiah Shore
31,168 PointsHow do I create a runnable JAR in Eclipse with included resource files?
I wrote a small program that reads data out of a SQL server database and writes the results of the query to CSV files. If the files don't exist it creates them. It then takes the CSV files and emails them to an email destination.
My program runs 100% fine inside of Eclipse. The CSV files are created and mailed correctly.
Here is the file structure:
-->project folder
|-->src
| |-->net.jeremiahshore
| |-->5 class files
| |-->2 images
|
|-->res
|--> ALL CSV files
I write to the files with the following method inside of a FileWriter class I created. The path variable is a string with the file path, e.g. "res/PROBLEM_REPORT.CSV".
public void writeToFile(String textLine) throws IOException {
FileWriter write = new FileWriter(path, append_to_file);
PrintWriter print_line = new PrintWriter(write);
print_line.println(textLine);
print_line.close();
}
The images in the main package are accessed by the following line; the images show up just fine when executing the runnable jar. This seems like a band-aid, and I feel like I should not be putting images in the package with class files.
Toolkit.getDefaultToolkit().getImage(getClass().getResource("example_image_name.gif"));
I then take these steps: Export > Runnable JAR File > Package required libraries into generated JAR
The problem I am running into is the code cannot find the files specified. I have done some research and everything is pointing that I need to do something with the classpath and that I should access the files in code via an InputStream. What's frustrating is that almost every resource available offers no explanation of what a classpath is/means and how I can compile a runnable JAR in eclipse and modify the classpath settings (most reference the command line only). Someone please help!
This is extremely dissatisfying when I was breaking ground with my code. It feels like there is no support or reliable knowledge on the Internet for this topic when it seems fundamental. Why is including resource files in a runnable JAR such a complex thing when almost every application imaginable could have files it relies on?
2 Answers

Craig Dennis
Treehouse TeacherHi Jeremiah,
JAR files are read-only. Does this Stack Overflow post help?
Let me know if you're still stuck!
Sounds like an awesome program! Good luck!
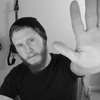
Jeremiah Shore
31,168 PointsI did not know that they were read only, I had only just heard of them from previous exposure to Java. Unfortunately that was not made obvious in any of my searching. Thanks for the tip :)