Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial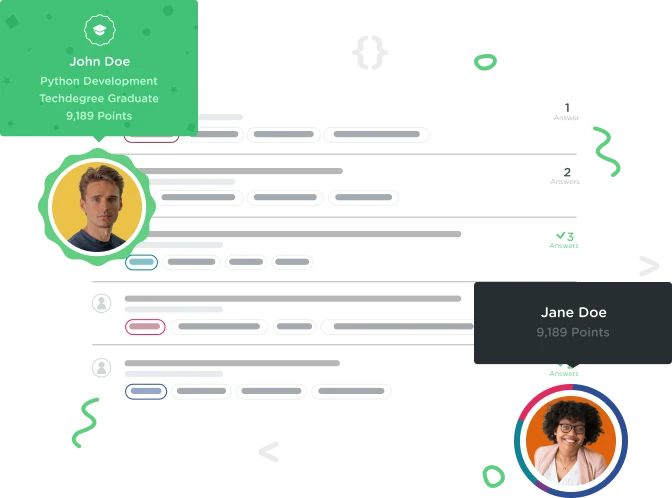

Sirin Srikier
7,671 PointsHow do I create a simple dropdown menu that goes to the url when you click the go button?
I'm trying to create a simple dropdown menu using <option> with the values of the url. When the user clicks on one of the options and clicks on the go button it will take you to the proper webpage (not a new tab). Here is my code so far.
<form> <select name="menu"> <option value="" >Please Choose...</option> <option value="http://www.google.com/">Google</option> <option value="http://www.youtube.com/">Youtube</option> <option value="http://www.pinterest.com/">Pinterest</option> </select> <form>
$( document ).ready(function() {
("option").val($(this).attr("href"));
('option').text($(this).text()); });
4 Answers
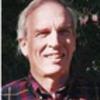
jcorum
71,830 PointsTreehouse has a course, jQuery Basics, that goes into a project like this in detail. Here's how Andrew does it there (slightly modified, as he creates the select):
$select = $("xxx") //where "xxx" is the id of your select (you'll need to add an id)
$select.change(function() {
//get value of select and navigate to that page
window.location = $select.val();
});
So, first you select the dropdown. Then, in a change() function you get the value of the selected option, which becomes the value of the select itself, and then use window.location to navigate there.
I forgot to add that here you don't need a button.
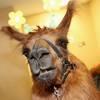
A X
12,842 PointsHi Sirin, Creating a simple JavaScript dropdown menu can be more involved than you think because creating it will involve integrating your JavaScript menu into the HMTL and CSS for your page. So you'll need to have code in all 3 that references each other. A resource to investigate further: http://www.w3schools.com/howto/howto_js_dropdown.asp
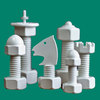
Steven Parker
230,274 PointsIt looks like you have a few issues yet:
- you still need to define a button
- you need to close your select
- your JavaScript additions to the options aren't necessary
- your JS code needs JQuery but your HTML doesn't appear to include it
Resolving those, plus changing menu to an ID (for convenience), and adding a small function and you might have something like this (and you don't even need JQuery):
<select id="menu">
<option value="" >Please Choose...</option>
<option value="http://www.google.com/">Google</option>
<option value="http://www.youtube.com/">Youtube</option>
<option value="http://www.pinterest.com/">Pinterest</option>
</select>
<button id="go" onclick="gotosite()">Go</button>
function gotosite() {
window.location = document.getElementById("menu").value; // JQuery: $("#menu").val();
}

Sirin Srikier
7,671 PointsThank you so much! I figured it out. I'll check out the jquery course as well.