Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial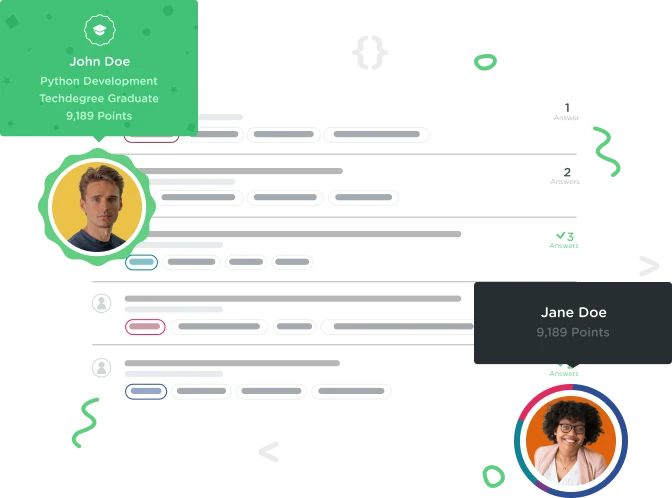

Gabri Chodosh
1,487 PointsHow do I create keys from all the words in a string? Do I combine a for loop and a packing/unpacking function?
I'm currently working on the Word Count challenge and I just don't know how to start -- I don't completely understand how these packing/unpacking functions work and I assume I'll need to use them to finish this challenge. I'm also not sure how to combine a for loop with a dict.keys() function.
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(string):
1 Answer
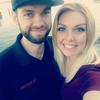
Bryan Reed
11,747 PointsI started by creating a blank dict I would later use as my return. Then creating a list of all the words in the string by splitting it on whitespace (making sure to lowercase the word first.
Now you can iterate through the list of words and by using the count() method (https://www.tutorialspoint.com/python/list_count.htm) you can .update() your blank dict with the proper keys and values you're iterating.
def word_count(string):
count_dict = {}
word_list = string.lower().split()
for word in word_list:
count_dict.update({word:word_list.count(word)})
return count_dict