Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial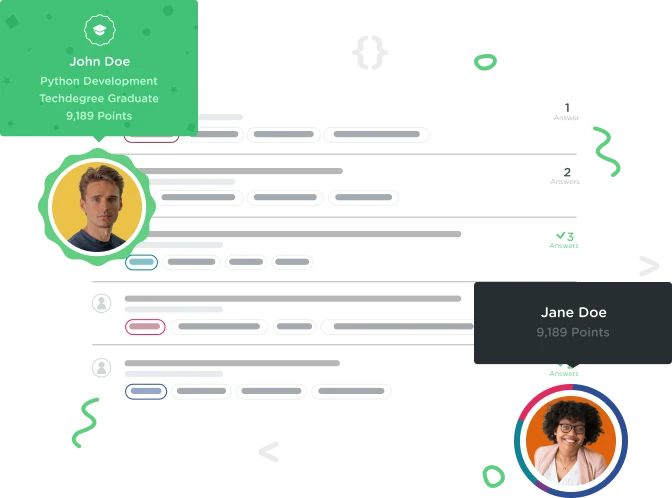

Zack Parr
4,091 PointsHow do I create the right members function?
I will admit for loops are a bit tricky for me, so I need a bail out when it comes to challenges like this
# You can check for dictionary membership using the
# "key in dict" syntax from lists.
### Example
# my_dict = {'apples': 1, 'bananas': 2, 'coconuts': 3}
# my_list = ['apples', 'coconuts', 'grapes', 'strawberries']
# members(my_dict, my_list) => 2
my_dict = {'red': 'f00', 'orange': 'f80', 'yellow': 'ff0', 'green': '0f0', 'blue': '00f', 'indigo': '40f', 'violet': '80f'}
my_list = ['red', 'green', 'blue']
def members(dic, lst):
venn = []
while True:
for key in dic:
for item in lst == key:
venn.append(item)
print (len(venn))
members(my_dict, my_list)
1 Answer
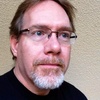
Chris Freeman
Treehouse Moderator 68,457 PointsYou are heading in the right direction. The for
loop runs a limited number of times due to the collection iterated over. This makes the while
unnecessary. Only one loop is needed since the other check can be an if
statement:
# Write a function named members that takes two arguments,
# a dictionary and a list of keys.
# Return a count of how many of the items in the list are also keys in the dictionary.
def members(dic, lst):
# initialize count
count = 0
# for key in dict
for key in dic:
# check if key is in list
if key in lst:
# increase count
count += 1
# return final count
return count
Additionally, It is more efficient to search for the relatively few list items within the dict keys, than to look for all of the dict keys in the small list.
def members(dic, lst):
# initialize count
count = 0
for item in lst:
if item in dic:
# increase count
count += 1
# return final count
return count
Freddy Venegas
1,226 PointsFreddy Venegas
1,226 PointsCould you help me clear something up. What would be the purpose of looking for and matching list items with dictionary key names, wouldn't it make more sense to match against the key value?
So, if my list was
list = ['fred', 'tom', 'jane']
and if my dictionary was
dict = {'member1':'fred', 'member2':'tom', 'member3':'jane'}
wouldn't it be more sensible to match as list items with key:values? A clarification would be much appreciated.