Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial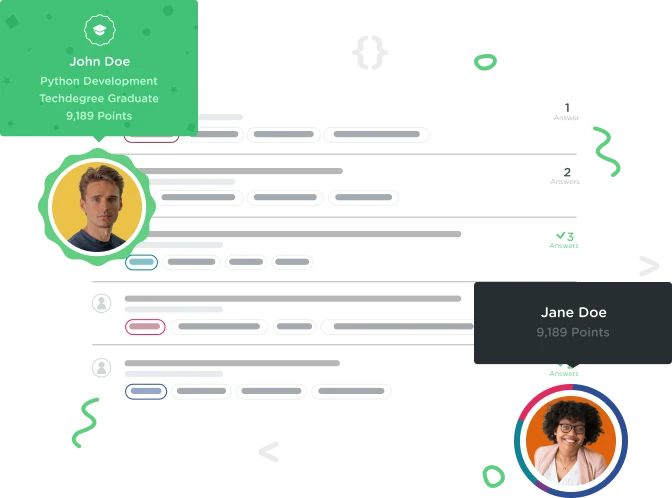

Moses Ong
889 PointsHow do I define Start to be falsey overtime?
Need some help to better understand how to solve this
import random
while True:
start = 5
random_num = random.randint(1,99)
def even_odd(num):
num = random_num
if num % 2 == 0:
print("{} is even".format(num))
else:
print("{} is odd".format(num))
start -= 1
# If % 2 is 0, the number is even.
# Since 0 is falsey, we have to invert it with not.
return not num % 2
2 Answers

Joe Beltramo
Courses Plus Student 22,191 PointsA loop that runs with while True
will never end because the value being checked by the while loop is True and not a condition. So to start, you will want to change your while loop to check by a condition that changes based on a value that is being mutated within the while loop. In this case start
while start > 0:
Here, we can now mutate the start variable's value to eventually make the start > 0
statement become false
Next you did not need to instantiate the start variable inside the while loop. You will want to keep that outside, otherwise you will be continually setting the value to 5 at the start of each loop iteration:
start = 5
while start > 0:
The predefined function even_odd
also does not need to reside inside the while loop, so simply, move it out and leave it unmodified
Lastly, inside the loops, the challenge would like you to decrement the start value by one, so that the loop can check the value of start
against 0
start -= 1
What you end up with is something like so
start - 5
while start > 0:
random_num = random.randint(1,99)
if even_odd(random_num):
# Here is where the {} is even statement goes
else:
# Here is where the {} is odd statement goes
start -= 1

Moses Ong
889 PointsHi Joe,
I'm grateful for the time you've taken to help me in this exercise
I've finally understood the concept.
I've updated the codes but it says I got the "Print" all wrong.
Is there something wrong with the updated code?
import random
start = 5
def even_odd(num):
return not num % 2
# Since 0 is falsey, we have to invert it with not.
while start > 0 :
random_num = random.randint(1,99)
if even_odd(random_num) % 2 == 0:
print("{} is even".format(random_num))
else:
print("{} is odd".format(random_num))
start -= 1

Moses Ong
889 PointsHey Joe, I've figured it out already.
import random
start = 5
def even_odd(num):
return not num % 2
# Since 0 is falsey, we have to invert it with not.
while start > 0 :
random_num = random.randint(1,99)
if even_odd(random_num) % 2 == 0:
print("{} is even".format(even_odd(random_num)))
else:
print("{} is odd".format(even_odd(random_num)))
start -= 1```