Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial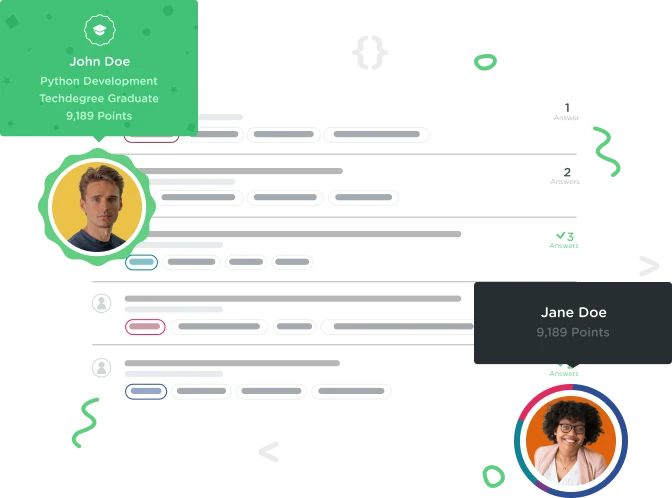

Geoff Parsons
11,679 PointsCould you post your attempt so we can comment on it? Just asking for the answer won't really help you much.
4 Answers
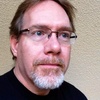
Chris Freeman
Treehouse Moderator 68,423 PointsThe challenge for Shopping List contain 4 parts.
Challenge Task 1 of 4 -- Create a variable named full_name
that holds your full name. Make another variable named name_list
that holds your full name as a list, split on the spaces. Don't create name_list
manually!
Step 1: Create a variable named full_name that holds your full name.
Variables are created when you first assign them a value. To assign a value to a variable place the name of the variable on the left-hand side of an equal sign ("=") and its new value on the right-hand side. Creating a variable called full_name
that has the value of your full name, say, "Aaron Williams", would look like this:
full_name = "Aaron Williams"
Step 2: Make another variable named name_list
that holds your full name as a list, split on the spaces.
From Step 1, you have your name as a string in the variable full_name
. Strings have a built-in method for splitting into lists call split()
. The default is to split on spaces. To call the split()
method use the "dot" syntax to add the method to the end of the variable containing the string. Assign the results of this to a new variable as was done in Step 1:
name_list = full_name.split()
Challenge Task 2 of 4 -- Create a variable named greeting_list
that's the string "Hi, I'm Treehouse" split on the spaces.
The newly created strings defined by quotes (") also have the same built-in method split()
. As in Task 1, assign string to the new variable and append the split()
method as below:
greeting_list = "Hi, I'm Treehouse".split()
Challenge Task 3 of 4 -- Almost done! Now, change "Treehouse"
in greeting_list
to the first item in your name_list
variable.
"Treehouse"
is the 3rd item in the greeting_list
. Using 0-based indexing, this item can be referenced as greeting_list[2]
. Your first name is the first item in your name_list. This can be referenced as name_list[0]
. Replacing Treehouse
with your first name can be like this:
greeting_list[2] = name_list[0]
Challenge Task 4 of 4 -- Great! Finally, make a variable named greeting
where you join greeting_list
back into a string, separated by spaces. You should end up with "Hi, I'm < your first name >"
as the value of greeting.
Strings also have a join()
method. The join()
method uses a base string as a joiner between items in a list. To join the updated greeting_list
into a string, use the join()
method on a string containing a single space like this:
greeting = " ".join(greeting_list)
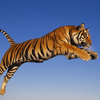
Diego Murray
2,515 PointsChallenge 4, the last line, will not pass. I tried it out in the Console of my workspace and it worked great. By the way, I really like your in depth description, very helpful.
It says "Bummer! Your name_list
's last item shouldn't be in your greeting
variable"
full_name = "Diego Murray"
name_list = (full_name).split(" ")
greeting_list = "Hi, I'm {}".format(full_name).split()
greeting_list[2] = name_list[0]
greeting = " ".join(greeting_list)
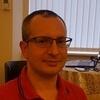
Giuseppe Elia Brandi
Front End Web Development Techdegree Graduate 69,630 PointsGood Day Chris, Do you think that this code is also functional? (see third line)
full_name = "Giuseppe Elia"
name_list = full_name.split(" ")
greeting_list = "Hi, I'm {}".format(name_list[0]).split(" ")
greeting = " ".join(greeting_list)
EDIT: formatting
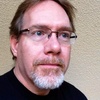
Chris Freeman
Treehouse Moderator 68,423 PointsIt's functional in the sense that you get the greeting_list
result needed. However, this code bypasses two elements of the challenge. First is that the code don't actually have a string with the word treehouse in it. The second is that the code is not substituting your first name for treehouse, instead you're creating greeting_list directly.
The last line, simply undoes the split(). Removing the split()
would eliminate the need to join()
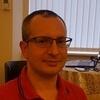
Giuseppe Elia Brandi
Front End Web Development Techdegree Graduate 69,630 PointsThanks Chris makes sense :)
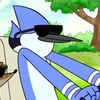
Zachary Fine
10,110 PointsIts funny, I am a developer whose worked with JavaScript, Ruby, Java and PHP but the "".join(greeting_list) stumped me. I remember in the video he said it was weird but thought nothing of it but now I totally agree. its soooooooooo weird.
William Li
Courses Plus Student 26,868 PointsWilliam Li
Courses Plus Student 26,868 Points??? how to do what?