Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial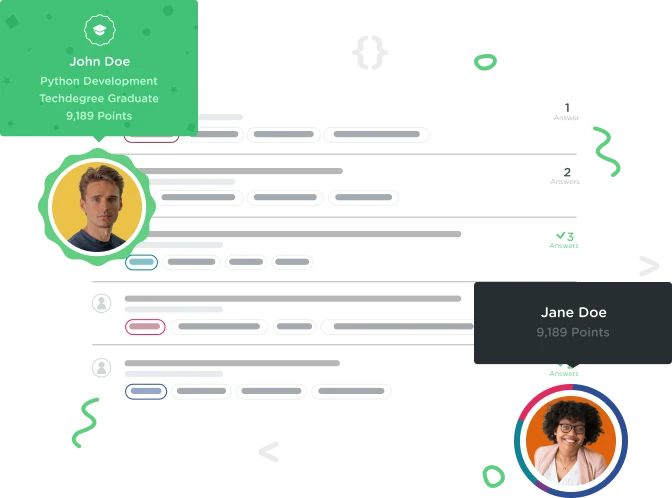

newtocoding
4,940 PointsHow do I do this challenge please? I'm stumped!
Hello, I'm trying to complete this challenge but I am completely mystified. I think I need to use fetchAll() somehow but I don't think it has been explained well enough for me to do this.
Can someone please explain how I can tackle this?
Here is the question:
The company you work for wants to send an email to its member base. They want the email to contain a complementary offer based on their membership level.
We've included a helper.php file that contain the connection to the database and give us a PDO object $db to use. This helper file also contains a function to send_offer. The send_offer function accepts the following arguments: $member_id, $email, $fullname, $level
In the current file, we've included the query to select the required items from the database. The results of the database query are stored in a PDOStatement object named $results. Loop through those results and pass them to the send_offer function.
Tip: we used this method in the last video
<?php
include "helper.php";
try {
$results = $db->query(
"SELECT member_id, email, fullname, level FROM members"
);
} catch (Exception $e) {
echo $e->getMessage();
}
//add code below this line
1 Answer
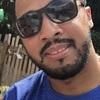
Jeffrey Howard
18,173 PointsRemember in the previous video the instructor shows us how to retrieve the data from the $results variable that has the query method assigned to it. You are correct that we need to use fetchAll(), which will organize the data stored in $results into an array.
First step is to type out $results->fetchAll() and assign this data to a new variable. The name of the variable can be whatever you like but we will choose $members.
$members = $results->fetchAll();
Next step is to use a foreach loop to pull the info needed to use inside of the send_offer function.
The last step is to pass the info into the send_offer function which will be inside the foreach loop. Remember the way we are taught to pull information out of associative arrays by using the array key to get the value. We know from the query statement that we are looking for the value of these keys "member_id, email, fullname, level".
For example: using $member['fullname'] inside of the foreach loop will display all of the $members full names.
Take a look at the full code below to see how we put it altogether.
//add code below this line
$members = $results->fetchAll();
foreach ($members as $member)
{
send_offer($member['member_id'],$member['email'],$member['fullname'],$member['level']);
}
Another video that helped is the lesson on PHP Arrays and Controls Structures / Associative Array. Gave me a quick refresher on how to handle associative arrays.
Hope this helps and happy coding.
newtocoding
4,940 Pointsnewtocoding
4,940 PointsThank you, this was extremely helpful! You're a lifesaver!