Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial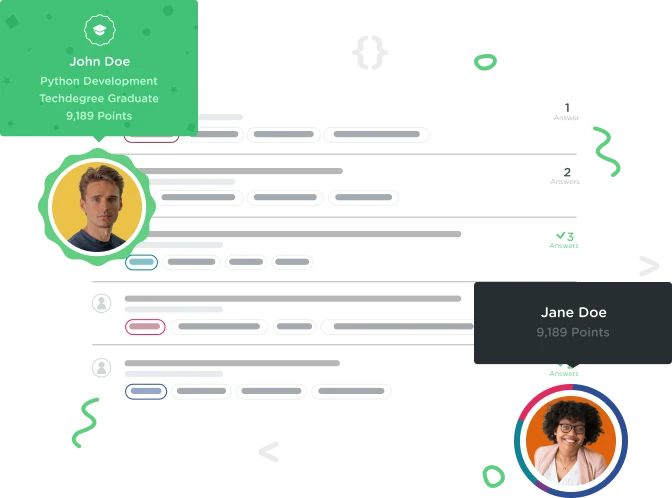
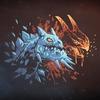
Dushyant Garg
772 PointsHow do i Do this im too confused
please help i dont seem to understand the question
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create a GoKart");
}
}
2 Answers
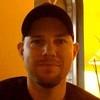
Jeremy Hill
29,567 PointsIn java, any time that you instantiate a new object you first write the class that you are creating an object from then you give it a name (usually something similar but not the same) and then you use the 'new' keyword, like this:
public class Example {
public static void main(String[] args) {
System.out.println("We are going to create an GoKart");
GoKart kart = new GoKart("red"); // this line creates a new GoKart object. I named it kart and it takes a single String argument ('red').
//Now we can use all of the GoKart methods on our GoKart object (kart) that we created.
}
}
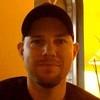
Jeremy Hill
29,567 PointsIn your GoKart class you added what is called a constructor that gets called every time a GoKart object gets created. This constructor basically initializes all of its member variables to what is passed in during the object instantiation. In your GoKart class your constructor should have looked something like this:
public class GoKart{
String mColor;
public GoKart(String color){ // this block of code is called the constructor; what distinguishes this as the constructor is that it doesn't have a return type and its name is the same as the class name.
//Whatever the parameters are is what needs to be passed in during object instantiation.
//So when you passed in a color in this challenge the constructor sets the member variable mColor to the color that you passed in.
mColor = color;
}
}
So if there is only one constructor with one String parameter then when you instantiate that object later it can only have exactly one String argument; if you try to pass in an additional argument it will throw an error. You can have several constructors as long as the arguments are not the same, otherwise it will confuse the compiler.
Dushyant Garg
772 PointsDushyant Garg
772 PointsThanks for the help !!