Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial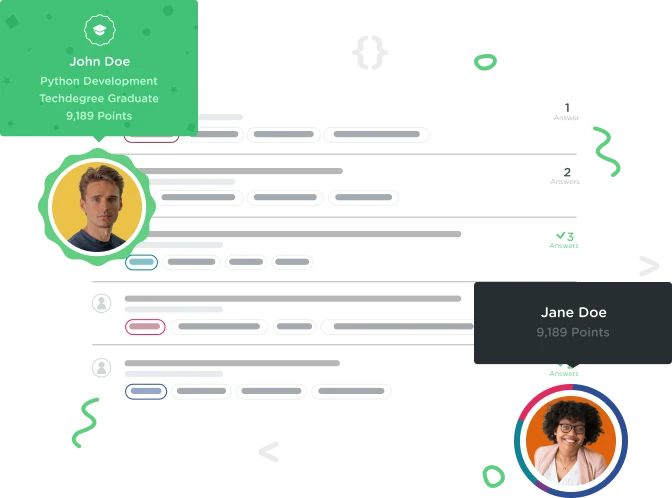
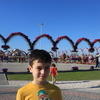
Farouk Charkas
1,957 PointsHow do I do this? What are they asking me to do?
How do I accomplish this challenge? I do not get the question.
1 Answer
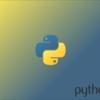
Kevin Faust
15,353 PointsHey Farouk,
This is the code we were given:
import android.os.Bundle;
public class FlightActivity extends Activity {
public int mFuelLevel = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_flight);
// Add your code below!
}
}
I'll try to make the instructions a bit easier to understand. Let's look back at the actual project we were building. We had a main activity and we linked it to another activity through the use of intents. Remember that? And we could pass data through activities by using putExtra() and receiving the string data by using getStringExtra().
In this challenge, basically it's saying that we already have a main activity and the code that we currently are on is the second activity. We want to receive the data we passed through our intent in the main activity.
In the onCreate() method, we simply create a new intent and then get the intent.
Intent intent = getIntent();
Does this look familiar?
For the second part of the challenge, we are now going to receive the intent we passed. However this time it is an int and NOT a string. Ben linked you to the intent documentation so you can see what parameters need to be passed in. We use the getIntExtra() method and pass it 2 values. The first is the key string which is "FUEL_LEVEL" as written in the instructions. The second is a default int value if no value is receive from our getIntExtra() method. The value we have to write is written in the instructions. We then store this int value in the mFuelLevel variable which is already initialized.
I attached the answer for your reference and in case you are still stumped:
import android.os.Bundle;
public class FlightActivity extends Activity {
public int mFuelLevel = 0;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_flight);
// Add your code below!
Intent intent = getIntent();
mFuelLevel = intent.getIntExtra("FUEL_LEVEL", -1);
}
}
Hope that helped and happy coding,
Kevin