Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial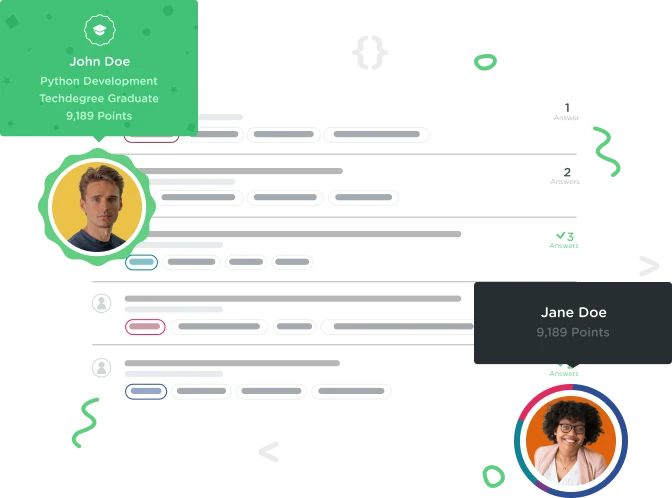

Kerri-Ann Bates
15,422 PointsHow do I echo just the key from an associative array?
For example:
$x = array('dog' => 'Spot', 'cat' => 'Bella');
Using the foreach
loop, how can I echo
'dog'.
<?php
$books["978-0743261690"] = "Gilgamesh";
$books["978-0060931957"] = "The Odyssey";
$books["978-0192840509"] = "Aesop's Fables";
$books["978-0520227040"] = "Mahabharta";
$books["978-0393320978"] = "Beowulf";
?><html>
<head>
<title>Five Great Books</title>
</head>
<body>
<h1>Five Great Books</h1>
<ul>
<?php foreach($books as $isbn => $book) { ?>
<li><?php echo $books . " (" . $isbn . ")"; ?></li>
<?php } ?>
</ul>
</body>
</html>
I get an error saying: Notice: Array to string conversion in index.php on line 17 Array (978-0743261690). Thank you.
<?php
$books["978-0743261690"] = "Gilgamesh";
$books["978-0060931957"] = "The Odyssey";
$books["978-0192840509"] = "Aesop's Fables";
$books["978-0520227040"] = "Mahabharta";
$books["978-0393320978"] = "Beowulf";
?><html>
<head>
<title>Five Great Books</title>
</head>
<body>
<h1>Five Great Books</h1>
<ul>
<?php foreach($books as $isbn => $book) { ?>
<li><?php echo $book; ?></li>
<?php } ?>
</ul>
</body>
</html>
1 Answer

Rich Donnellan
Treehouse Moderator 27,708 PointsYou have an error in the foreach
loop:
<li><?php echo $books . " (" . $isbn . ")"; ?></li>
$books
is the array, and you want to echo
out a single $book
. I also find it easier to concatenate using the double quotes; much easier to read, IMO (and still passes the challenge). Anything variables inside are interpreted.
<li><?= "$var1 ($var2)"; ?></li> // using the echo shorthand (valid in PHP 5.4 and up)
Kerri-Ann Bates
15,422 PointsKerri-Ann Bates
15,422 PointsAahhh right, the error is from $books. I missed that detail. Thank you! And also thanks for the tip using the echo shorthand.