Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial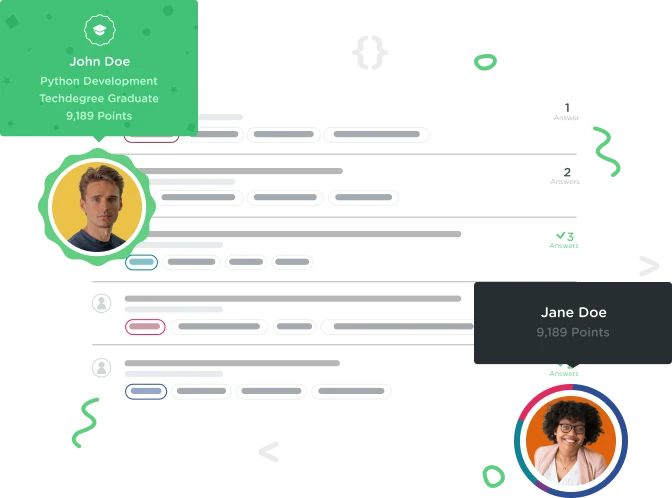
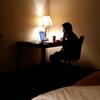
Michael Bianchi
2,488 PointsHow do I execute a function that takes in an Object's parameters in JavaScript?
I have a constructor function that looks like this:
anArray = ["red","blue","green"]; var dataSet = function (int1, int2, int3); var setOne = new dataSet(1, 2, 3);
var firstFunction = function(){ for(var x = 0; x < anArray.length; x++){ // apply second function to anArray[x] if the user types an element // in that array. } }
2 Answers
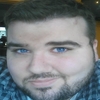
Marcus Parsons
15,719 PointsHey Michael,
All you need to do to be able to pass in parameters to a function is put at least one placeholder variable (you can name it practically whatever you want) inside the parenthesis when you create the function like so:
var myFunction = function (placeholder) {
}
In the above example, placeholder
is a placeholder variable that will take the place of whatever you pass into the function. So, if you pass in anArray
like you have above:
myFunction(anArray);
When anArray
gets passed into myFunction
, the variable placeholder
becomes a reference to anArray
. That means you can now iterate over the array placeholder, and then do something like log the contents of the array to the console:
anArray = ["red","blue","green"];
var firstFunction = function (array) {
for (var x = 0; x < array.length; x += 1) {
console.log(array[x]);
}
}
//put the contents of "anArray" into the placeholder "array" which is named in firstFunction
firstFunction(anArray);
There are other ways to pass in arguments to a function without declaring placeholder variables such as using the arguments
keyword, but I won't go over any of those right now because it is likely to confuse you at this point in time.
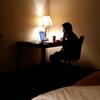
Michael Bianchi
2,488 PointsThank you very much! I was stuck on this for days now. I voted you best answer. And thanks for syntax highlighting my crap, Mr. Erdrag (lol)!
erdragerdragsson
Courses Plus Student 5,887 Pointserdragerdragsson
Courses Plus Student 5,887 Pointsi formatted the code so it has syntax highlight