Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial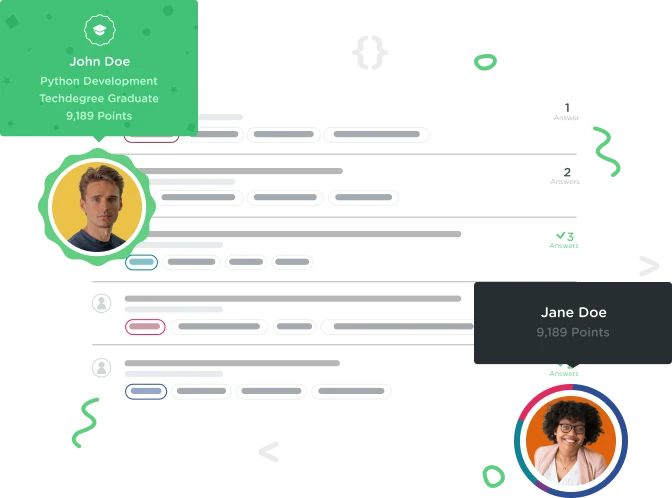

ziv brodie
1,348 PointsHow do I fix this error?
For some reason I keep on having this error even though I followed the instructions.
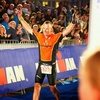
Steve Hunter
57,712 PointsEdited to hide your API key & to highlight the code block.

ziv brodie
1,348 PointsWait im not sure what you mean, do you want me to highlight the place where the error is occuring?
2 Answers
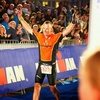
Steve Hunter
57,712 PointsNo - I amended your post so that the code block looks like it does now and I also removed your API key from your post as this is private to you and your app.
The error itself is an odd one. Something is being treated as a JSON Object when it is in fact a String.
If I had this code working in my environment, I would be questioning this line:
String JsonData = response.body().toString();
... as I think the last method should be .string()
making the line look alternatively like:
String JsonData = response.body().string();
But I don't have it running so I can't test that little hunch.
Steve.
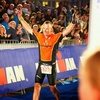
Steve Hunter
57,712 PointsI'd also amend the variable name to jsonData
to comply with naming conventions.
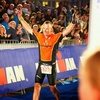
Steve Hunter
57,712 PointsAh, yes! If you look at your Log
below that line, you've not been D.R.Y. but have used the different method there.
I think that's the correct method, so the first line should use string()
not toString()
and then you can use the variable jsonData
(as amended) in the Log
so as not to have that code repeated.

ziv brodie
1,348 PointsBut why would those things cause an error? aren't they just preference?
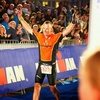
Steve Hunter
57,712 PointsThe toString()
method returns your object in string format - not the response, the whole object. Where string()
returns your response as a string, which is what you're after.

ziv brodie
1,348 PointsBut if i am only calling toString() on a response then wouldnt it just return the response in string format? or does toString() specifically for converting objects to string format?
ziv brodie
1,348 Pointsziv brodie
1,348 PointsHere is the code:
here's the error: 07-21 01:37:10.644 20859-20908/com.example.android.stormy E/MainActivityοΉ Caught exception: org.json.JSONException: Value com.squareup.okhttp.internal.http.RealResponseBody@423e87e0 of type java.lang.String cannot be converted to JSONObject at org.json.JSON.typeMismatch(JSON.java:111) at org.json.JSONObject.<init>(JSONObject.java:158) at org.json.JSONObject.<init>(JSONObject.java:171) at com.example.android.stormy.MainActivity.getCurrentDetails(MainActivity.java:73) at com.example.android.stormy.MainActivity.access$100(MainActivity.java:22) at com.example.android.stormy.MainActivity$1.onResponse(MainActivity.java:54) at com.squareup.okhttp.Call$AsyncCall.execute(Call.java:170) at com.squareup.okhttp.internal.NamedRunnable.run(NamedRunnable.java:33) at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1076) at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:569) at java.lang.Thread.run(Thread.java:856)