Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial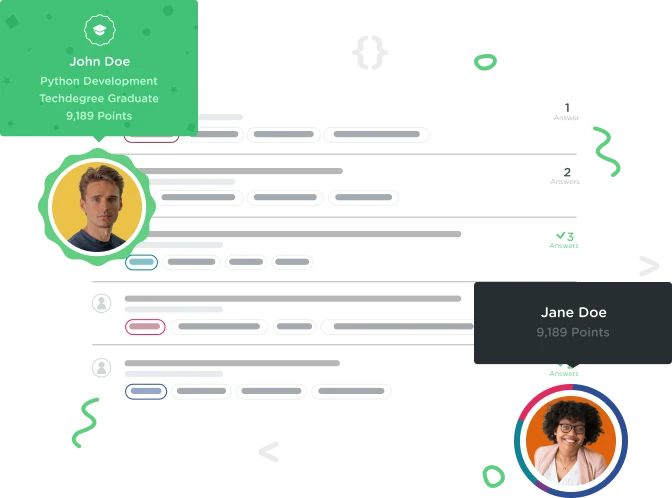

helmi al kindy
1,371 PointsHow do I get an array to become a string ?
In this exercise I have to print out a string but it comes out as a list. It says I have to use the .join() but when I do I get an error that says AttributeError: 'list' object has no attribute 'join' What does that mean ? How do I use the join method for this exercise ?
full_name = 'jon doe'
name_list = full_name.split()
greeting_list = "Hi, I'm Treehouse".split()
greeting_list[2] = name_list[0]
greeting = str(greeting_list)
2 Answers
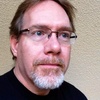
Chris Freeman
Treehouse Moderator 68,423 PointsIt is not obvious how to use join
. join()
is a string method and can not be used on a list, as you found out. Using help(str.join)
for help:
join(...)
S.join(iterable) -> str
Return a string which is the concatenation of the strings in the
iterable. The separator between elements is S.
This means, use the string separator defined as S
to join the items in the iterable. So to join the items in greeting_list
with spaces, S
would be a string with a single space: " ":
greeting = " ".join(greeting_list)

helmi al kindy
1,371 PointsSo let's assume x = [1, 2, 3] Would x be the object and 1, 2 and 3 be its attributes ?
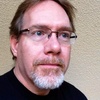
Chris Freeman
Treehouse Moderator 68,423 PointsNot exactly. The items in the list x
such as the number 1 are objects in themselves which are referenced by the list, but are not attributes of x
. If you want to see the attributes of a specific list, such as x, use the python statement dir(x)
helmi al kindy
1,371 Pointshelmi al kindy
1,371 PointsOK thanks, but what is an attribute error ?
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsThis might be a little too deep of an explanation....
Attributes are the items within a class. This includes methods of an object class such as upper() for strings:
str.upper()
and append() for lists:list.append()
. As you start to create class objects you will learn more on this.You may often hear "everything in python is an object". Well, all of the items within an object are attributes of that object. When trying to interpret your code, python looks up the attributes you reference within each object. So when trying to use
join
on a list, it looks up the methodjoin
within the attributes of thelist
object. Because it does not exist for a list, there is no join attribute. Thus the error returned by python is an "AttributeError".