Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial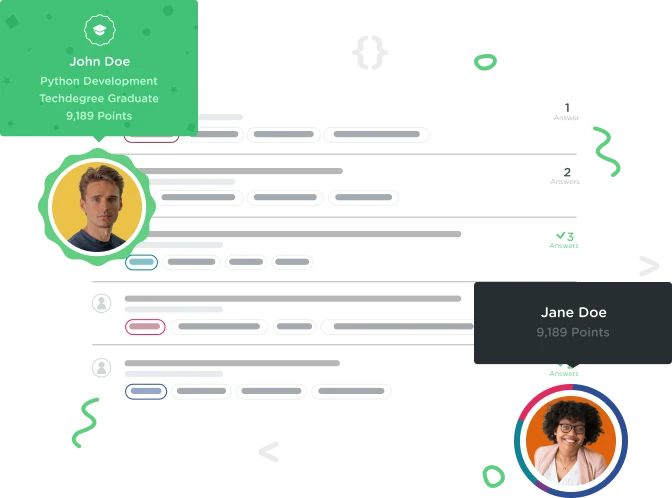

Ryan Scharfer
Courses Plus Student 12,537 PointsHow do I get Flickr to respond JSON that I can use?
I am trying to use plain Javascript for an AJAX request. The code below works if I remove the JSON parse bit. As it is, it doesn't. :(
I think the problem is Flickr is not returning valid JSON, instead giving me the responseText in a syntax like: JSONFlickrApi(validJSON).
var flickrAPI = "";
flickrAPI += "https://api.flickr.com/services/rest/?";
flickrAPI += "&method=flickr.photos.search";
flickrAPI += "&api_key=9012151640d5486e63780579ff3b9cae";
flickrAPI += "&tags=Altuve";
flickrAPI += "&per_page=5";
flickrAPI += "&page=1";
flickrAPI += "&format=json";
function myFunction(){
var xhr= new XMLHttpRequest();
xhr.open("GET",flickrAPI,true); //
xhr.send();
xhr.onreadystatechange=function(){
if(xhr.readyState==4 && xhr.status==200) {
var myArray = JSON.parse(xhr.responseText);
console.log(myArray);
}
};
};
How do I parse the responseText that is returned into JS that I can use, or alternatively, make sure that valid JSON is returned? I know this is probably explained somewhere in Dave's videos, but posting in the forum seemed easier than trying to find where this is discussed. : )
1 Answer

Matt West
14,545 PointsHi Ryan,
You're encountering an error here because the Flickr API is returning JSONP and not standard JSON. (You can read more about JSONP here: http://en.wikipedia.org/wiki/JSONP)
If you want the Flickr API to respond with standard JSON you need to add the nojsoncallback
parameter to your query string and set it's value to 1
.
&nojsoncallback=1
The updated code would look something like the following:
var flickrAPI = "";
flickrAPI += "https://api.flickr.com/services/rest/?";
flickrAPI += "&method=flickr.photos.search";
flickrAPI += "&api_key=9012151640d5486e63780579ff3b9cae";
flickrAPI += "&tags=Altuve";
flickrAPI += "&per_page=5";
flickrAPI += "&page=1";
flickrAPI += "&format=json";
// UPDATE: Request plain old JSON
flickrAPI += "&nojsoncallback=1";
function myFunction() {
var xhr= new XMLHttpRequest();
xhr.open("GET",flickrAPI,true); //
xhr.send();
xhr.onreadystatechange=function(){
if(xhr.readyState==4 && xhr.status==200) {
var myArray = JSON.parse(xhr.responseText);
console.log(myArray.photos);
}
};
};
myFunction();
Hope this helps! :)
Ryan Scharfer
Courses Plus Student 12,537 PointsRyan Scharfer
Courses Plus Student 12,537 PointsHi, Matt! Thank you. And this was all clearly explained in Dave's videos. It just goes to show you that you have to try something out yourself and apply the concepts yourself if you want to get a deeper understanding of the subject material. : )