Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial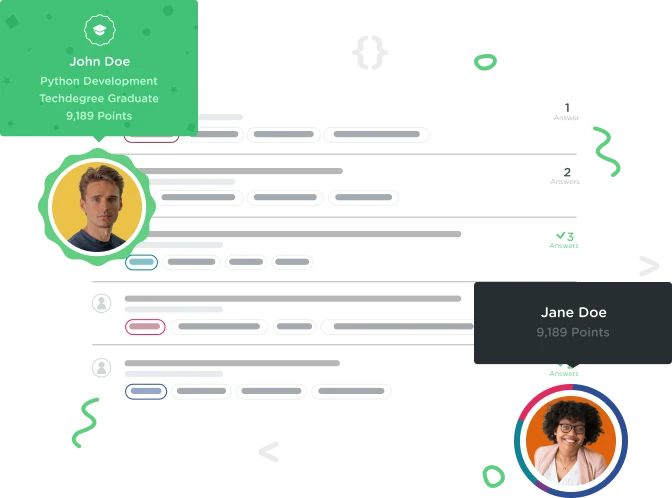
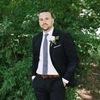
Cory Cromer
5,171 PointsHow do I get rid of this error in the init method?
I reformatted the currentWeatherViewModel so it reformats the icon stored property from a string value to a UIImage instance. I did this through an extension using a func; however, when I use the func in the init method, I get an error reading, "'self' used before all stored properties are initialized." I do not know how to fix this. Any ideas?
import Foundation
import UIKit
//This ViewModel formats CurrentWeather for the ViewController through a custom init method
struct CurrentWeatherViewModel {
let temperature: String
let humidity: String
let percipProbability: String
let summary: String
let icon: UIImage
init(model: CurrentWeather) {
let formattedTemperature = Int(model.temperature)
self.temperature = "\(formattedTemperature)ΒΊ"
let formattedHumidity = Int(model.humidtiy * 100)
self.humidity = "\(formattedHumidity)%"
let formattedPercipProbability = Int(model.percipProbability * 100)
self.percipProbability = "\(formattedPercipProbability)%"
self.summary = model.summary
let formattedIcon = convertIcon(named: model.icon)
self.icon = formattedIcon
}
}
extension CurrentWeatherViewModel {
func convertIcon(named: String) -> UIImage {
switch named {
case "clear-day": return #imageLiteral(resourceName: "clear-day")
case "clear-night": return #imageLiteral(resourceName: "clear-night")
case "rain": return #imageLiteral(resourceName: "rain")
case "snow": return #imageLiteral(resourceName: "snow")
case "sleet": return #imageLiteral(resourceName: "sleet")
case "wind": return #imageLiteral(resourceName: "wind")
case "fog": return #imageLiteral(resourceName: "fog")
case "cloudy": return #imageLiteral(resourceName: "cloudy")
case "partly-cloudy-day": return #imageLiteral(resourceName: "partly-cloudy")
case "partly-cloudy-night": return #imageLiteral(resourceName: "cloudy-night")
default: return #imageLiteral(resourceName: "default")
}
}
}
***error occurs under the convertIcon(named: model.icon)
code