Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial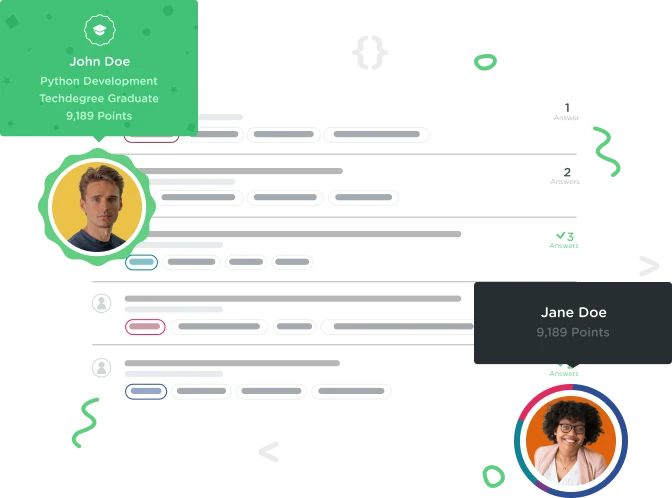

Luke Telford
2,931 PointsHow do i get the first 4 and last 4 items of a list?
So i have created a function and i need to get it to return the first and last 4 items of the list, how do i do this? i though returning [:4:-4] would work but it doesn't as this just returns 1
def first_4(list):
return list[:4]
def odds(list):
return list[1::2]
def first_and_last_4(list):
return list[:4:]
8 Answers

Cristian Altin
12,165 PointsI can only help you with a hint and an explanation because giving you the full answer would not be helpful for your learning.
To access the last for elements you need to do something like this: mylist[-something:]
Hint: Now one way of solving it is to ".extend()" the other result or "add" it. ;-)
Let me make a quick summary of how this feature works. mylist[from : to(excluded) : step(optional)]
Suppose you have this array:
mylist = ['a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm']
Then:
mylist[0] is 'a'
mylist[0:1] is also 'a'
mylist[1:1] is [] (empty) because "to" excludes the index you enter so it cannot be the same as "from"
If "from" is 2 it will start at index 2. Since indexes start at 0 (0,1,2,3,...) it will be the 3rd item of the array.
If "to" is 3 it will stop at index 2. So mylist[2:3] only gives the value of index 2.
mylist[5:] will give you the entries from index 5 to the end > ['f', 'g', 'h', 'i', 'j', 'k', 'l', 'm']
mylist[:5] will give you the entries from the beginning to index 4 (since 5 will be excluded) > ['a', 'b', 'c', 'd', 'e']
mylist[-1:] will give you the last entry in the list because negative numbers start at the end
mylist[-4:] will start at 4th from last and end at the last entry > ['j', 'k', 'l', 'm']
mylist[5:-4] will start at index 5 and end at 4th from last > ['f', 'g', 'h', 'i']
Now for the optional argument:
mylist[::] is the same as mylist[:] and mylist[::1] since it will give the whole array
mylist[::2] has step = 2 so it will take one every 2 from the array > ['a', 'c', 'e', 'g', 'i', 'k', 'm']
mylist[1::2] will start taking every two entries from index 1 (second entry) > ['b', 'd', 'f', 'h', 'j', 'l']
mylist[::-1] has step = -1 so it will output the whole array but in reverse order > ['m', 'l', 'k', 'j', 'i', 'h', 'g', 'f', 'e', 'd', 'c', 'b', 'a']
mylist[::-2] will take one every two starting at the end in reverse order > ['m', 'k', 'i', 'g', 'e', 'c', 'a']
mylist[-8:1:-1] when having a negative step (-1) your first argument will start at the "right side" and go down to the left. In this example remember that "to" is excluded but this time being in reverse order it will stop at index 2. > ['f', 'e', 'd', 'c']
Nyasha Chawanda
7,946 Pointsfirst create a variable containing the first four values then using the extend() function extend the tlats four values with the first value the return the variable extended as follows:
def rt (lis): first4 = lis[:4] last4 = lis[-4:] last4.extend(first4) return (last4)
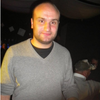
Vittorio Somaschini
33,371 PointsHello Luke.
I honestly don't know if there is a way to do it directly the way you have tried to do.
I have tried to do the code challenge again and I simply used the plus sign between the two slices (first 4 and last four).
I hope I made it clear, if still unsure we can have a look at the code together.
Maybe someone knows how to do it in a single step though.
Vittorio

Luke Telford
2,931 PointsHello Vittorio,
Your help is more understandable to me but I'm not quite sure about how to go about adding two slices together. I have tried to use the .extend method how i think it should done but still no luck, this is what i came up with. Obviously i would like to be able to do it alone so if you can explain how i should use the .extend or how to add two slices together that would be grand!
def first_4(list): return list[:4]
def odds(list): return list[1::2]
def last_4(list): return list[:-4]
def first_and_last_4(): return first_4.extend(last_4)

Cristian Altin
12,165 PointsLuke, what Vittorio suggested is to concatenate using + instead of .extend() which is what I did to solve the exercise but requires more intuition.
If you study my post more carefully you will see how the selector for slicing lists works. In your latest code you try to get the last for items with list[:-4] but by looking at my post you can see that mylist[from : to(excluded) : step(optional)]. This means that you are taking all elements from the beginning to the fourth-to-last element excluded. By doing list[-4:] instead you will be getting all the elements from the fourth-to-last to the end which is what you want.
Pay close attention to the fact that you are often forgetting to send the list to a function when calling it and that first_and_last_4(something missing here) is not receiving any.
In the end you can just return something like list[:] + list[:] where you set them accordingly. ;-)

Luke Telford
2,931 PointsHello Christian,
I under stand the whole slicing and stepping with dictionaries it was just the sheer amount that you have wrote that kinda threw me off, i know how to get the first 4 and last 4 times using two functions it was just the concatenating them i am struggling with, i will give your advice another read and see if i can do it. Thanks for all the help though

Samuel Adjei
Full Stack JavaScript Techdegree Student 4,789 PointsHey guys, I am finding an issue with this challenge...
My code is as follows: def first_and_last_4(x): first_4 = x[:4] last_4 = x[-4:] return (first_4.extend(last_4))
However, I get the error: Bummer: Couldn't find first_4
.
Any ideas?

Leonid Zadonaisky
4,054 PointsThis kind of funny :) just don't delete the first function you created "first_4" and create the new one "first_4_and_last_4" below

Sagar Jain
2,140 PointsSolution - def first_and_last_4(x): first_4= x[:4] print first_4 last_4 = x[-4:] print last_4 print (first_4+last_4)
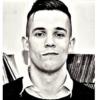
Daniel JAHENY
1,912 PointsThis is how I brute-forced it, not very elegant but it did the trick:
def first_4 (s): l = list(s) f = l[:4] return(f)
def first_and_last_4(s): l = list(s) f = l[-4:] g = l[:4] h = (g + f) return (h)

piotr korczowski
9,986 PointsThis is my solution:
def first_4(list): return list[:4]
def first_and_last_4(list): return(list[:4] + list[-4:])
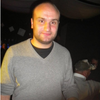
Vittorio Somaschini
33,371 PointsHello Luke.
I only wrote a little in my reply as I thought you were actually pretty close: you know how to get the first 4 element and you probably know how to get the last 4 elements.
I just concatenated putting the + sign between these 2 slices, something like:
return "first 4 elements" + "last for elements"
Where between the quotes we need the code to get those elements.
;)
Luke Telford
2,931 PointsLuke Telford
2,931 PointsI finally managed to do it! i couldn't do it by passing the first_4 and last_4 functions so instead i just got the function to return 2 lists and concatenated them :) thank you for all your help!