Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial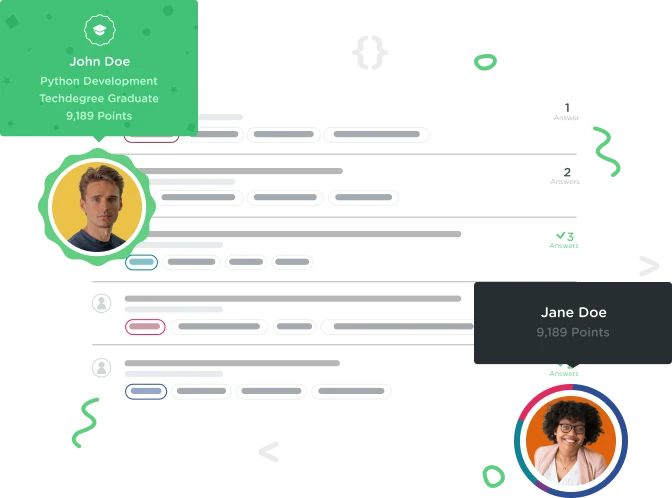

Gokul Nishanth
Courses Plus Student 581 PointsHow do I get the value one dice?
Great! Let's make one more scoring method! Create a score_yatzy method. If there are five dice with the same value, return 50. Otherwise, return 0.
class YatzyScoresheet:
def score_ones(self, hand):
return sum(hand.ones)
def _score_set(self, hand, set_size):
scores = [0]
for worth, count in hand._sets.items():
if count == set_size:
scores.append(worth*set_size)
return max(scores)
def score_one_pair(self, hand):
return self._score_set(hand, 2)
def score_chance(self, hand):
return sum(hand)
2 Answers

Paul Bentham
24,090 PointsThis Stack Overflow answer was really helpful for me on this one: http://stackoverflow.com/questions/3787908/python-determine-if-all-items-of-a-list-are-the-same-item
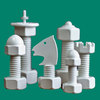
Steven Parker
229,786 Points
I got your message.
This might be a good place for a loop. By iterating through the array items, you could get one at a time and compare it to the first. If any are different, return 0. But if the loop finishes they must all be the same so return 50.
If you are familiar with comprehensions you could use that instead of a loop and make the code more compact.
Paul Bentham
24,090 PointsPaul Bentham
24,090 PointsRemember the values of your dice are stored in the list "hand" so hand[0], hand[1], hand[2] all need to be the same, how would you check for that? Checkout the Stack Overflow question.
Travis Bailey
13,675 PointsTravis Bailey
13,675 PointsI used your article, and it eventually led me to the correct answer. However, one approach the article mentions does not work. Could you explain why?
After using a different approach to check equality mentioned in the article it passed the challenge, but the above code did not.
Steven Parker
229,786 PointsSteven Parker
229,786 PointsIt seems like it should, since sets can only contain unique items. So if you create a set from a list of items all the same, the set size should be 1.
Travis Bailey
13,675 PointsTravis Bailey
13,675 PointsI found the issues thanks to Kennteth Love.
Issue 1: Die has no hash defined and set members must be hashable. Kenneth updated the challenge so Die now has hash defined.
Issue 2: Need to name method 'score_yatzy()' not 'score_chance()' ....woops