Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial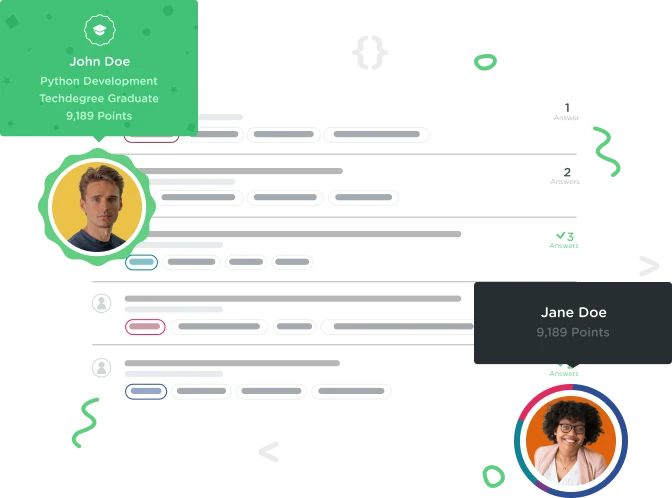
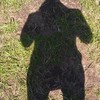
Nathan McElwain
4,575 PointsHow do I get this to work?
I've worked on this a bunch of different ways, but I just can't quite figure out how to get the right outcome,
dicts = [
{'name': 'Michelangelo',
'food': 'PIZZA'},
{'name': 'Garfield',
'food': 'lasanga'},
{'name': 'Walter',
'food': 'pancakes'},
{'name': 'Galactus',
'food': 'worlds'}
]
string = "Hi, I'm {name} and I love to eat {food}!"
def string_factory(dicts, string):
newList = []
newDict = dicts.pop()
a = string.format(**newDict)
newList.append(a)
return newList
3 Answers
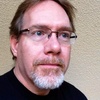
Chris Freeman
Treehouse Moderator 68,454 PointsYou need to add a loop to iterate over the dict_list. Something like:
def string_factory(orders, format_string):
formatted = []
for order in orders:
formatted.append(format_string.format(**order))
return formatted
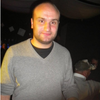
Vittorio Somaschini
33,371 PointsHello Nathan.
I am struggling understanding what you wanted to do with your code. Especially the pop method line as I can't really see the need for it.
I have moved back to the code challenge and solved it this way:
I have typed the same code as yours till newList= [], but if I got it right in python we should not use camelCase but something like string_list = [] as per conventions (I think).
After that I have made a loop to go through all the dictionaries in dicts, and just appended the formatted string each time to the string_list we have just initialized. I see you got this part as you wrote a very similar line of code : string.format(**newDict)
After that I have returned the string_list: make sure indentation is good, because the return statement in this case has to have same indentation as the for in loop.
I am going to paste my code below, it is not a very good practice I think as you may want to try and solve it by yourself now before having a look at it, but since I think it is important to understand the solution I came up with, I am going to paste it anyway, so
DO NOT SCROLL DOWN IF YOU WANT TO TRY AGAIN BY YOURSELF!
def string_factory(dicts, string):
string_list = []
for dictionary in dicts:
string_list.append(string.format(**dictionary))
return string_list
I hope this helped.
Let me know if any other questions.
Vittorio
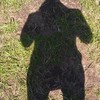
Nathan McElwain
4,575 PointsThank you both. I was having a hard time figuring out how to iterate through the list.