Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial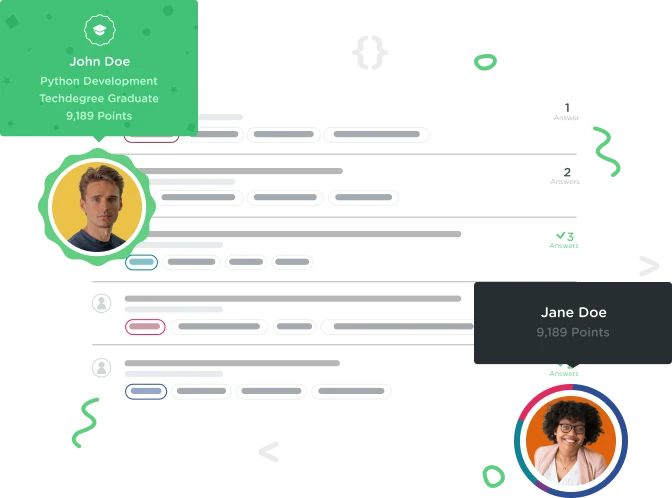
jordans
1,609 PointsHow do I go through two-dimensional arrays and create a new array containing the largest numbers of each array?
I've been working on this exercise for two long now and I can't seem to figure out how to do this. Basically, there's supposed to be a two-dimensional array with 4 inner arrays and 4 numbers inside those arrays. I'm trying to grab the highest number of all four arrays and create a new array containing those numbers.
The four arrays I'm using are [4, 5, 1, 3], [13, 27, 18, 26], [32, 35, 37, 39], [1000, 1001, 857, 1]. So, with those in mind the final output should look like this: [5, 27, 39, 1001].
I feel like I'm using too many for loops, but I can't figure out any other way. Thanks.
var array = ([
[4, 5, 1, 3],
[13, 27, 18, 26],
[32, 35, 37, 39],
[1000, 1001, 857, 1]
]);
for (var i = 0; i < 1; i++) {
var arrays = array[i];
for (var j = 0; j < array.length; j++) {
var largest = arrays[0];
for (var k = 0; k <= largest; k++) {
if (arrays[k] > largest) {
var largest = arrays[k];
}
}
console.log(largest);
}
};
1 Answer

Daniel Newman
Courses Plus Student 10,715 Pointsvar array = ([
[4, 5, 1, 3],
[13, 27, 18, 26],
[32, 35, 37, 39],
[1000, 1001, 857, 1]
]);
var largestArray = [] // You'll see the reason of this var later
// Why don't you use array.length instead of
// for (var i = 0; i < 1; i++) {
for (var i = 0; i < array.length; i++) {
var arrays = array[i];
// Here it is - first mistake you did
// array length is 1 and you need arrayS you've just defined
// for (var j = 0; j < array.length; j++) {
for (var j = 0; j < arrays.length; j++) {
var largest = arrays[0];
// It's useless loop, we already in great loop inside arrayS[]
/*
for (var k = 0; k <= largest; k++) {
if (arrays[k] > largest) {
var largest = arrays[k];
}
}
*/
if( arrays[j] > largest ) {
largest = arrays[j];
}
console.log(largest);
// It will console each time whenever we redefine the largest value or not
// We showing up the largest of array element but
// we can store it in array two different ways:
// largestArray.push( largest ) and
// largestArray[i] = largest
// I'll prefer the first one
}
// Here it is. We've fetch whole inner arraS and find the largest one
largestArray.push( largest );
};
// Let see what we've got:
console.log(largestArray)
But if you really need to store ARRAY of Largest number we can push it as largestArray
And without comments:
var array = ([
[4, 5, 1, 3],
[13, 27, 18, 26],
[32, 35, 37, 39],
[1000, 1001, 857, 1]
]);
var largestArray = [];
for (var i = 0; i < array.length; i++) {
var arrays = array[i];
for (var j = 0; j < arrays.length; j++) {
var largest = arrays[0];
if (arrays[j] > largest) {
largest = arrays[j];
}
}
largestArray.push(largest);
};
console.log(largestArray);
laurahenry
12,665 Pointslaurahenry
12,665 PointsThis solution does not work. I tested it and it returns [4, 26, 39, 1000]. It should return [4, 27, 39, 1001]