Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial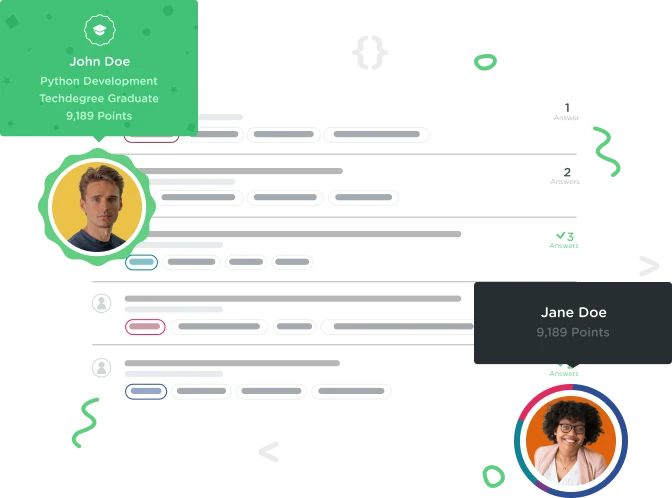
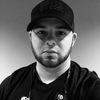
Michael Fazekas
3,070 Pointshow do I import a const array/object from another file in React?
I am trying to export my ProductData from my Data.js file in react and be able to import anywhere in my components. so far this is what I am and nothing is showing up even though I don't have any errors or warnings. when I console.log(ProductData) it returns all my objects.
const ProductData = [
{
id: 1,
company: "GOOGLE",
title: "Google Pixel-Black",
img: "image/product-1.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 499,
},
{
id: 2,
company: "SAMSUNG",
title: "Samsung Galaxy-White",
img: "image/product-2.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 799,
},
{
id: 3,
company: "HTC",
title: "HTC-Black",
img: "image/product-3.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 399,
},
{
id: 4,
company: "HTC",
title: "HTC-White",
img: "image/product-4.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 425,
},
{
id: 5,
company: "HTC",
title: "HTC-Black/Red",
img: "image/product-5.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 499,
},
{
id: 6,
company: "APPLE",
title: "Iphone 3GS",
img: "image/product-6.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 349,
},
{
id: 7,
company: "APPLE",
title: "Iphone 6s",
img: "image/product-7.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 799,
},
{
id: 8,
company: "APPLE",
title: "Repair Screen",
img: "image/product-8.png",
info:
"Lorem ipsum dolor amet offal butcher quinoa sustainable gastropub, echo park actually green juice sriracha paleo. Brooklyn sriracha semiotics, DIY coloring book mixtape craft beer sartorial hella blue bottle. Tote bag wolf authentic try-hard put a bird on it mumblecore. Unicorn lumbersexual master cleanse blog hella VHS, vaporware sartorial church-key cardigan single-origin coffee lo-fi organic asymmetrical. Taxidermy semiotics celiac stumptown scenester normcore, ethical helvetica photo booth gentrify.",
inCart: false,
count: 0,
total: 0,
price: 149,
},
{
siteName: "COMPhone Store",
},
];
export default ProductData;
NAVBAR trying to render siteName to H1 to make sure im doing it correctly... //apparently not..
import React from "react";
import ProductData from "../Data";
console.log(ProductData);
const Navbar = ({ siteName }) => {
return (
<div>
<h1>{ProductData.siteName}</h1>
</div>
);
};
export default Navbar;
1 Answer
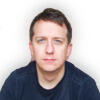
Phil Livermore
11,018 PointsProductData is an array of objects so you would need to specify the index:
<h1>{ProductData[8].siteName}</h1>