Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial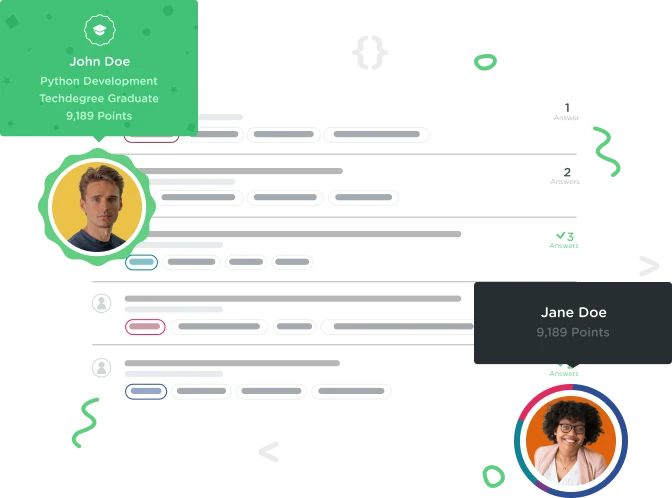

Daniel Tadros
4,633 PointsHow do I initialize the property "tag"
struct Tag { let name: String }
struct Post { var title: String var author: String var tag: Tag init(title: String, author: String, tag: Tag) { self.title = title self.author = author self.tag = Tag(name: name) }
}
let somePost = Post(title: "p", author: "p", tag: Tag)
struct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
}
2 Answers

jakubhirner
14,784 PointsStructs in swift have default initializers. You can initialise it the same way as you did with the post, it wood look like this:
struct Tag {
let name: String
}
struct Post {
var title: String
var author: String
var tag: Tag
}
let tag = Tag(name: "some tag 1")
let firstPost = Post(title: "First Post", author: "author 1", tag: tag)
// you can also do it directly
let secondPost = Post(title: "Second Post", author: "author 2", tag: Tag(name: "some tag 2"))
This is what the initialiser really looks like:
struct Tag {
let name: String
init(name: String) {
self.name = name
}
}
struct Post {
var title: String
var author: String
var tag: Tag
init(title: String, author: String, tag: tag) {
self.title = title
self.author = author
self.tag = tag
}
}
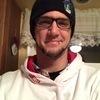
Ryan Sady
20,594 PointsLike stated above, Structs in Swift have 'default' initializers. If you don't declare an initializer within your struct the compiler does it for you based on your declared properties. If you have optional properties or want to do certain logic in your initializer you can manually declare one and perform your logic and initialize your properties.