Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial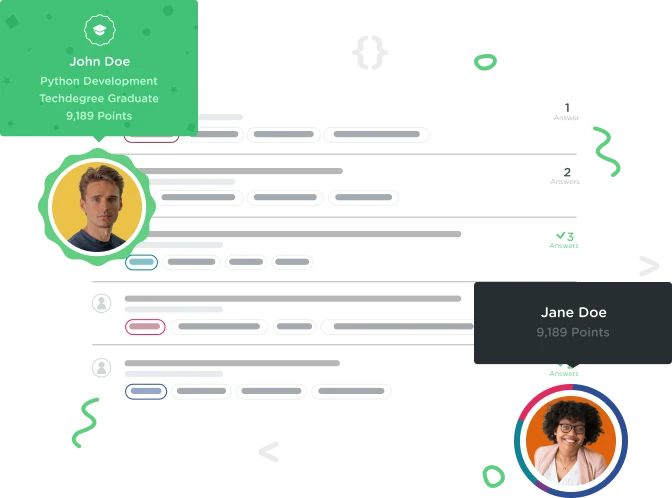
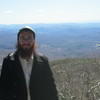
Yosef Fastow
18,526 PointsHow do I make a list of instances?
I want to make a list of 5 random monsters in the monster game to fight different types of monsters every game. But once you defeated one type of monster and have that same type again it start's off with 0 hit_points and dies the next turn. I think that it doesn't saves it in the list as a new instance but as the same instance. I think I could solve it by specifying 5 new instances with its own name and add it to the list but I sure there a easier way to add new instances by doing a loop. How would one do that?
Here is my code now:
def monsters_list(self):
self.monsters_li = []
num = 0
monster_list = [
Goblin(),
Troll(),
Dragon()
]
while num < 5:
monster = random.choice(monster_list)
self.monsters_li.append(monster)
num += 1
return self.monsters_li
def setup(self):
self.player = Character()
self.monsters = self.monsters_list()
self.monster = self.get_next_monster()
1 Answer
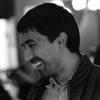
Martin Cornejo Saavedra
18,132 PointsThe problem is that, you are calling the same instances of goblin, troll and dragon again and again. You have to create new instances when you append a random choice. I'd try this:
def monsters_list(self):
self.monsters_li = []
num = 0
monster_list = [
Goblin, #we remove the parentheses
Troll,
Dragon
]
while num < 5:
monster = random.choice(monster_list)() #here we initialize a new
#monster with parentheses
self.monsters_li.append(monster)
num += 1
return self.monsters_li
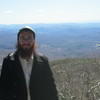
Yosef Fastow
18,526 PointsThanks! I tried it out and it worked perfectly. I knew it was something simple like that.
Yosef Fastow
18,526 PointsYosef Fastow
18,526 PointsI tried to call each one on its own and I got the same problem.