Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial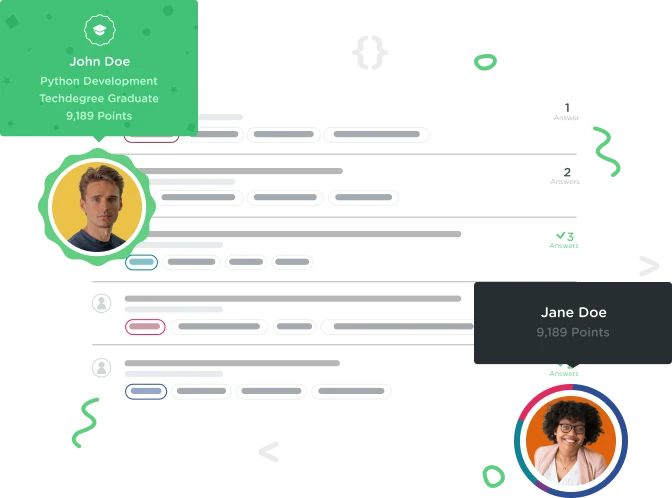
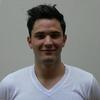
Szabolcs Varga
Courses Plus Student 662 PointsHow do i make a Parse query for a username that was added to a UITextField?
I have a UITextfield called searchField, a UIButton called searchButton and a Table View. I want to query for the username that was written into the UITextField and display the result in the table view.
It would be amazing, if somebody could help me to figure out the right way. I tried to do this way without any success:
'''objective-c
-
(void)viewDidLoad { [super viewDidLoad];
mainArray = [[NSArray alloc] initWithObjects:@"User", nil];
}
-(NSInteger) tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section { return [mainArray count]; }
-(UITableViewCell *) tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
UITableViewCell *cell = [tableView dequeueReusableCellWithIdentifier:@"thisCell"]; cell.textLabel.text = [mainArray objectAtIndex:indexPath.row]; return cell; }
-
(IBAction)searchButton:(id)sender { NSString *searchResult = [self.searchField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
PFQuery *query = [PFUser query]; [query whereKey:@"username" equalTo:@"searchResult"]; [query includeKey:@"username"]; [query getFirstObjectInBackgroundWithBlock:^(PFObject *object, NSError *error) { if (object) { PFUser *user = (PFUser *)object; NSLog(@"Username: %@", user[@"username"]); } }];'''
-
3 Answers

Unsubscribed User
6,125 PointsÖrülök, hogy segíthettem. :) A továbbiak in English: You just need replace the type of mainArray in the header to NSMutableArray and the initialization in the viewDidLoad to
mainArray = [[NSMutableArray alloc] initWithObjects:@"User", nil];
Here is the useful link for difference between NSArray and NSMutableArray: http://stackoverflow.com/questions/1345215/difference-between-nsarray-and-nsmutablearray

Unsubscribed User
6,125 PointsYou can use PFQueryTableViewController instead of UITableViewController, here you find the documentation: https://parse.com/docs/ios_guide#ui-tables/iOS (use the @"_User" for parseClassName property)
But if you still use UITableViewController, this code is working for me: ([query includeKey:] method is just used, when you need fetch related objects, documentation: https://www.parse.com/docs/ios_guide#objects-pointers/iOS or http://blog.parse.com/2011/12/06/queries-for-relational-data/)
- (IBAction)searchButton:(id)sender {
NSString *searchResult = [self.searchField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
PFQuery *query = [PFUser query];
[query whereKey:@"username" equalTo:searchResult];
[query getFirstObjectInBackgroundWithBlock:^(PFObject *object, NSError *error) {
if (object) {
PFUser *user = (PFUser *)object;
NSLog(@"Username: %@", user.username);
if (mainArray.count > 0) {
[mainArray replaceObjectAtIndex:0 withObject:user.username];
} else {
[mainArray addObject:user.username];
}
[self.tableView reloadData];
}
}];
}
I hope that I helped.
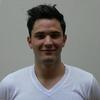
Szabolcs Varga
Courses Plus Student 662 PointsKoszonom szepen, hogy szantal idot a dologra! Kiraly, hogy pont belebotlottam egy magyarba :). A lenyegi reszt irom inkabb angolul, hogy masok is ertsek ;).
I have to use a normal Table View because PFQTVC can't be a subclass of a UIViewController . I tried the code and got this error : No visible @interface for 'NSArray' declares the selector 'addObject:'. Is it because mainArray is an instance variable in this block? (I declared it in the .h and also allocated in the viewDidLoad) Or any idea?

Unsubscribed User
6,125 PointsBocs, véletlenül nem erre nyomtam reply-t, de lentebb megtalálod a válaszokat. :)

Unsubscribed User
6,125 Pointsor if you want to use the NSArray, you just reinitialize the array with the username.
- (IBAction)searchButton:(id)sender {
NSString *searchResult = [self.searchField.text stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]];
PFQuery *query = [PFUser query];
[query whereKey:@"username" equalTo:searchResult];
[query getFirstObjectInBackgroundWithBlock:^(PFObject *object, NSError *error) {
if (object) {
PFUser *user = (PFUser *)object;
NSLog(@"Username: %@", user.username);
mainArray = [[NSArray alloc] initWithObjects:user.username, nil];
[self.tableView reloadData];
}
}];
}
Szabolcs Varga
Courses Plus Student 662 PointsSzabolcs Varga
Courses Plus Student 662 PointsMukodik! Szuper, koszi szepen. Kb. tegnap reggel ota szenvedtem a dologgal. Legkozelebb itt kezdem egybol :))