Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial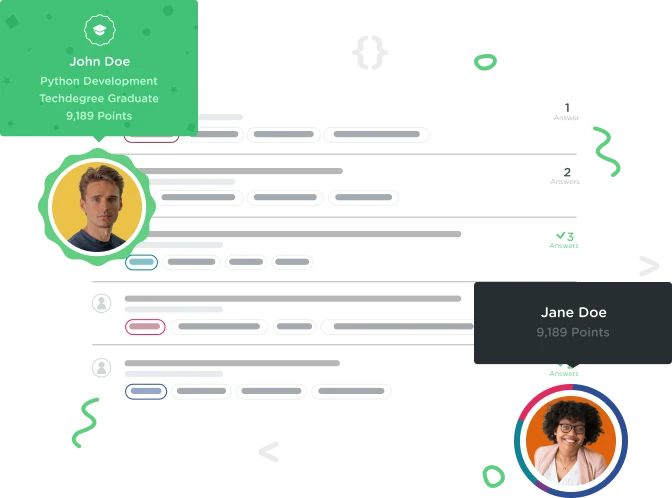
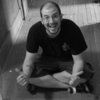
Mark Shaw
10,449 PointsHow do I make an event happen when pressing a *specific* key?
I'd like to call a function when the user pushes the enter key. I know there are things like keydown event listener but I need this to work only with the enter key.
Basically, I have a text input field and a function that stores the users input into a variable and then does something with the variable but I have no idea how to make the event trigger when the user presses the enter key, and only the enter key.
Any help would be greatly appreciated!
3 Answers
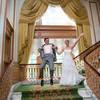
Colin Bell
29,679 PointsIf you're using jQuery, you can use keydown()
$(document).keydown(function(keyPressed) {
if (keyPressed.keyCode == 38) {
$("body").css("background-color","tomato");
}
});
If the key pushed has a keyCode of 38 (which is the up key), then turn the background of the body to "tomato".
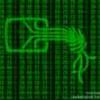
Andre Kettschau
20,054 Pointskeydown listener is the right approach, you just have to check, which key was actually pressed.
You can get the keycode from the event with e.keyCode.
The enter-key has Code 13 :)
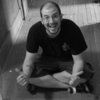
Mark Shaw
10,449 PointsThis is what I have.
userChoice.addEventListener('keydown', flip);
How do I make the keydown argument only work for enter? For instance, what argument would I pass instead of keydown?
I'm sorry, I'm sure the answer is obvious but I can't seem to find it anywhere I've looked. It's driving me crazy!
Mark Shaw
10,449 PointsMark Shaw
10,449 PointsThanks for the help! jQuery was my fall back plan but I really wanted to be able to do it without jQuery. I'm still on the fence about jQuery. I love it (well, the little I know of it.) but I feel like I need to get a better understanding of 'pure' javascript before I start to rely too much on things like jQuery.
Colin Bell
29,679 PointsColin Bell
29,679 PointsWhat about something like this then:
Mark Shaw
10,449 PointsMark Shaw
10,449 PointsSo I could use the function to check the key press and call the function I wanted to call initially within the if block? The fact that I didn't think of that makes me upset haha! That's so simple. I think I was just looking for a complicated answer. I'll give it a shot.
Thanks so much for the help!
edit: It worked brilliantly! Thanks again.