Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial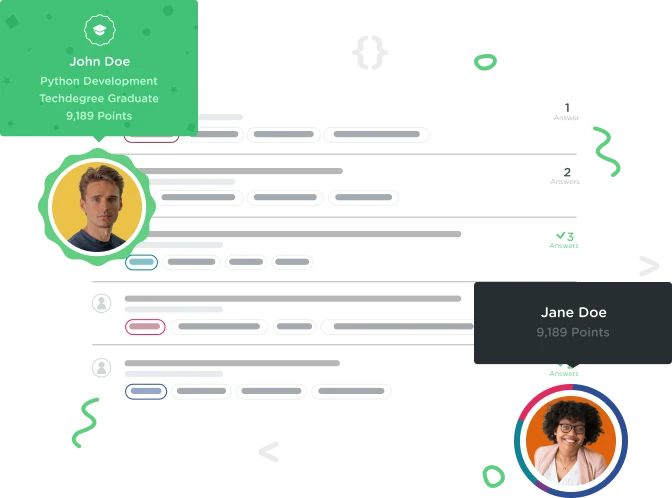

Anders Axelsen
3,471 PointsHow do I make code print("You win! The word was {}.".format(secret_word))?
My code doesn't print "You Win!...". Any idea of what's wrong? :) Here is my code:
import random
# make list of words
words = [
'apple'
'banana'
'orange'
'coconut'
'strawberry'
'lime'
'grapefruit'
'lemon'
'kumquat'
'blueberry'
'melon'
]
while True:
start = input("Press enter/return to start, or enter Q to quit")
if start.lower() == q:
break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
# draw guessed letters, spaces and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
# take guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter, kammerat!")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}.".format(secret_word))
else:
bad_guesses.append(guess)
else:
print("You didn't guess it! My secret word was {}.".format(secret_word))
# draw guessed letters and strikes
# print out win/lose
3 Answers
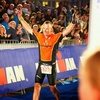
Steve Hunter
57,712 PointsWhen I run your code I get an error at this line, because you need to highlight q as being a char, not a variable.
if start.lower() == q:
change to:
if start.lower() == 'q':
I then get a huge list of all your words array to guess!
Guess a letter: i
applebananaorangecoconutstrawberrylimegrapefruitlemonkumquatblueberrymelon
Strikes: 2/7
But, no, I get no completed message either. I shall do more investigation!
Steve.

Hadi Khalili
7,969 PointsI have the same problem. After looking over each line of code again and again. I suspected that the repeating letters in the word is the issue, I think only one instance of the letter is appended while both are printed to the screen. Then I included words with no repeating letters and it worked. So my suspicion was correct. I think it might be taken care of in the next videos.
Now to answer your other Question:
Do you agree, that the last else responds to the first if ?
else belongs to the immediate if statement of matching indentation. First if statement that checks guess length not equal to 1 has no else block. The second if statement that checks guess in secret_word has an else block which appends to bad_guesses.
Hope all these helps

Anders Axelsen
3,471 PointsBoy, Hadi, it helps big time!
I find repeating the simpler things make room for the more advanced ideas of syntax.
Thank you.
Kindly, Anders.

Jonathan Camacho
1,504 PointsWow, it does work now. How did it work for Kenneth?

Anders Axelsen
3,471 PointsHi Steve. I just received your reply. The else should correspond to the first if in your 3rd code example, unless I am mistaken.
Thank you very much for helping!
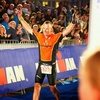
Steve Hunter
57,712 PointsHi Anders,
The else
I was talking about is the last two lines of your whole code block. That's not the code I posted; that is where I think you should handle this outcome.
Anyway, I think we've progressed well. I suggest you test your code and see if there's new changes required. We can take that from there.
Steve.

Anders Axelsen
3,471 PointsHi again Steve.
I changed the 'q'
and inserted the commas. I am pretty sure, the last else
is supposed to respond to the first if
in the first while
-loop.
Reflecting upon this brings about for me a better understanding of the overall syntax.
Do you agree, that the last else
responds to the first if
?
Anders
P.P.S.: It works :-) - changing the position of the else
gives me a SyntaxError. Thanks for good feedback.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsSeparate your list with commas; that fixes the long phrase issue.
I now get told I won & lost!
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNot sure what the last two lines are doing; where's the
if
for theelse
?Maybe handle the
bad_guess
being full in theelse
part here:Steve.