Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial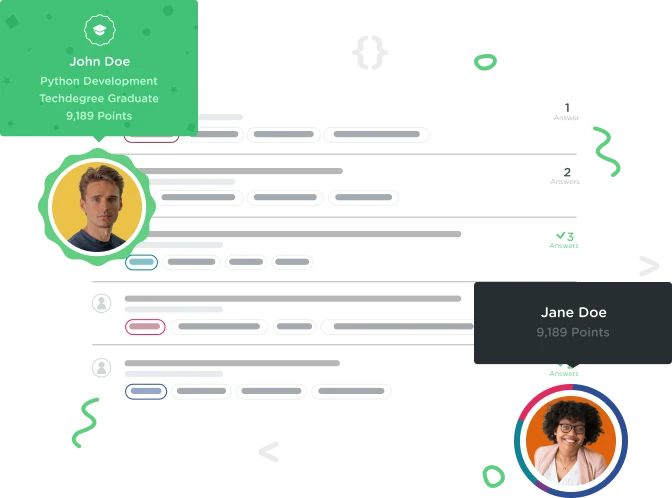

Alex Zhou
11,943 PointsHow do I make "for i in range(2, a)" iterate through everything before the else statement?
I'm trying to find the sum of all primes below a given number (Ex: Sum of all primes if N = 10 would be 17 because 2+3+5+7=17). However, I messed up somewhere and I don't know where. I did some trial and error and when i changed N from 15 to 16 counter went up by 15 even though it should've stayed the same. I think it's because the else statement triggered first before "for i in range(2, a):" went through everything, but I'm not sure. Can someone help me fix this please? Thanks!
N=16
counter=0
for a in range(2, N):
for i in range(2, a):
if a % i == 0:
break
else:
counter+=a
break
print(counter)
1 Answer
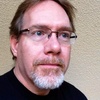
Chris Freeman
Treehouse Moderator 68,454 PointsHey Alex Zhou, your code is not behaving as you might think.
I added print statements for N=10 and got the following output:
a= 3 ; i= 2
adding a 3 break
a= 4 ; i= 2
2 divides into 4 break
a= 5 ; i= 2
adding a 5 break
a= 6 ; i= 2
2 divides into 6 break
a= 7 ; i= 2
adding a 7 break
a= 8 ; i= 2
2 divides into 8 break
a= 9 ; i= 2
adding a 9 break
24
Seems i
never progresses beyond 2.
If the else
is move out such that it aligns with the for
loop instead of the if
statement, and remove the break
in the else
block, the results seem correct:
adding a 2 break
a= 3 ; i= 2
adding a 3 break
a= 4 ; i= 2
2 divides into 4 break
a= 5 ; i= 2
a= 5 ; i= 3
a= 5 ; i= 4
adding a 5 break
a= 6 ; i= 2
2 divides into 6 break
a= 7 ; i= 2
a= 7 ; i= 3
a= 7 ; i= 4
a= 7 ; i= 5
a= 7 ; i= 6
adding a 7 break
a= 8 ; i= 2
2 divides into 8 break
a= 9 ; i= 2
a= 9 ; i= 3
3 divides into 9 break
17
with this change, N=15 and N=16 yield the same result: 41
adding a 2 break
a= 3 ; i= 2
adding a 3 break
a= 4 ; i= 2
2 divides into 4 break
a= 5 ; i= 2
a= 5 ; i= 3
a= 5 ; i= 4
adding a 5 break
a= 6 ; i= 2
2 divides into 6 break
a= 7 ; i= 2
a= 7 ; i= 3
a= 7 ; i= 4
a= 7 ; i= 5
a= 7 ; i= 6
adding a 7 break
a= 8 ; i= 2
2 divides into 8 break
a= 9 ; i= 2
a= 9 ; i= 3
3 divides into 9 break
a= 10 ; i= 2
2 divides into 10 break
a= 11 ; i= 2
a= 11 ; i= 3
a= 11 ; i= 4
a= 11 ; i= 5
a= 11 ; i= 6
a= 11 ; i= 7
a= 11 ; i= 8
a= 11 ; i= 9
a= 11 ; i= 10
adding a 11 break
a= 12 ; i= 2
2 divides into 12 break
a= 13 ; i= 2
a= 13 ; i= 3
a= 13 ; i= 4
a= 13 ; i= 5
a= 13 ; i= 6
a= 13 ; i= 7
a= 13 ; i= 8
a= 13 ; i= 9
a= 13 ; i= 10
a= 13 ; i= 11
a= 13 ; i= 12
adding a 13 break
a= 14 ; i= 2
2 divides into 14 break
a= 15 ; i= 2
a= 15 ; i= 3
3 divides into 15 break
41
See [Python docs on else clauses on loops (https://docs.python.org/3/tutorial/controlflow.html#break-and-continue-statements-and-else-clauses-on-loops) for more insight on why this works.
Also, you may want to use for a in range(2, N+1):
so that you add N if it happens to be prime.
Post back if you need more help. Good luck!!!