Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial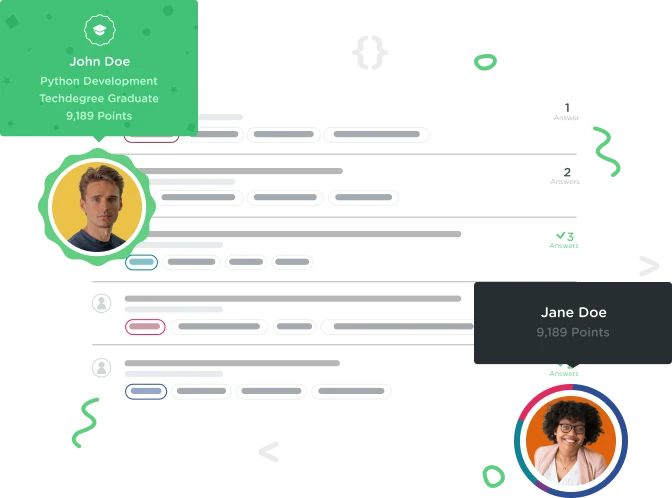

Oti Oritsejafor
3,281 PointsHow do I make my monster move?
It says 'steps' is not defined in line 93(indicated in the code). Also, the monster is M which I have made visible on the board. Thanks in advance
import random
BOARD = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
monster = random.choice(BOARD)
start = random.choice(BOARD)
door = random.choice(BOARD)
#If player, door or monster are random, do it all over again.
if monster == door or monster == start or door == start:
return get_locations()
return monster, door, start
def move_player(player, move):
x, y = player
if move == 'LEFT':
y -= 1
elif move == 'RIGHT':
y += 1
elif move == 'UP':
x -= 1
elif move == 'DOWN':
x +=1
else:
print("That move doesn't exist PLEASE.")
return x, y
def move_monster(monster, steps):
x, y = monster
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
steps = random.choice(moves)
if steps == 'LEFT':
y -= 1
elif steps == 'RIGHT':
y += 1
elif steps == 'UP':
x -= 1
elif steps == 'DOWN':
x +=1
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if player[1] == 0:
moves.remove('LEFT')
if player[1] == 2:
moves.remove('RIGHT')
if player[0] == 0:
moves.remove('UP')
if player[0] == 2:
moves.remove('DOWN')
return moves
def draw_map(player, monster):
print('_ _ _')
tile = '|{}'
for idx, cell in enumerate(BOARD):
if idx in [0, 1, 3, 4, 6, 7]:
if cell == player:
print(tile.format("X"), end ='')
elif cell == monster:
print(tile.format("M"), end = '')
else:
print(tile.format("_"), end ='')
else:
if cell == player:
print(tile.format("X|"))
elif cell == monster:
print(tile.format("M|"))
else:
print(tile.format("_|"))
monster, player, door = get_locations()
print("Welcome to the dungeon! *evil laugh*")
while True:
moves = get_moves(player)
draw_map(player, monster)
print("You are currently in room {}".format(player)) #fill in with player position
print("You can move {}".format(moves)) # fill in with available positions
print("Enter 'GIVEUP' to quit")
move = input("> ")
move = move.upper()
move_monster(monster, steps) LINE 93
if move == "GIVEUP":
print("Giving up, you wait sadly for the Beast to find you. It does, and makes a tasty meal of you...")
print("You lose.")
break
if move in moves:
player = move_player(player, move)
else:
print("Walls are hard, stop walking into them!")
continue
if player == door:
print("You narrowly escaped the beast and escaped")
print("You win!")
break
elif player == monster:
print("The beast found you!")
print("You lose!")
print("Game over")
break
# If it's a good move, change the player's position
# If it's a bad move, don't change anything
# If the new position is the door, they win!
# If the new positon is the Beast's, they lose!
# Otherwise, continue
1 Answer

Gavin Ralston
28,770 Pointswhile True:
moves = get_moves(player)
draw_map(player, monster)
print("You are currently in room {}".format(player)) #fill in with player position
print("You can move {}".format(moves)) # fill in with available positions
print("Enter 'GIVEUP' to quit")
move = input("> ")
move = move.upper()
move_monster(monster, steps)
You're getting a name error because you haven't assigned a value to the variable "steps", so when you pass it into the move_monster() function, the interpreter doesn't have any idea what to do.
It looks like you copied that from the method definition. Make sure you find the value/variable you really want to pass to that function.
Also, please notice that in your function definition, you never use the steps parameter--you overwrite it with a random choice from the monster's moves list. Maybe you don't need that there at all?
def move_monster(monster, steps):
x, y = monster
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
steps = random.choice(moves) # <--- right there you assign a value to 'steps' without ever using the value passed in...
if steps == 'LEFT':
### ... you never really use 'steps' for anything other than storing some information local to the function.

Oti Oritsejafor
3,281 PointsThanks!
Oti Oritsejafor
3,281 PointsOti Oritsejafor
3,281 PointsSorry, it actually says this
Traceback (most recent call last):
File "dungeon2.py", line 93, in <module>
move_monster(monster, steps)
NameError: name 'steps' is not defined