Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial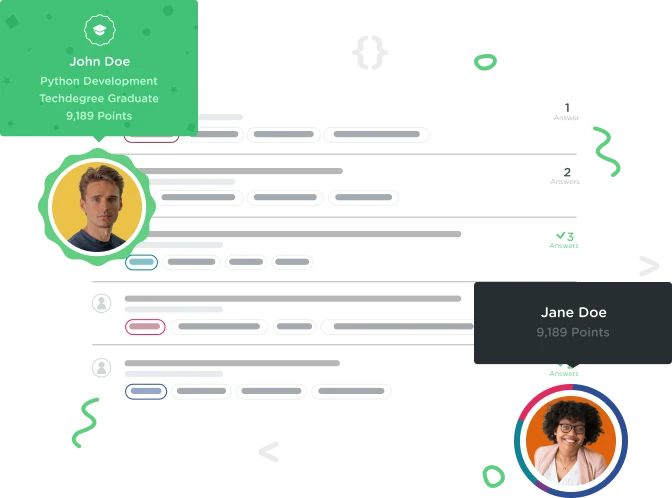

Gary Gibson
5,011 PointsHow do I make the spaces appear between the words in the secret phrase of this hangman game?
I've had lots of fun tweaking this hangman game so that the player can do things like reveal the vowels (at the cost of five tries) or attempt to solve the puzzle in one turn.
But I cannot seem to get a blank space between the dashes to represent a space between words. So "vampire bite" shows as ------------ instead of ------- ----.
The player CAN enter a space if they need to either as a guess or when they type in the phrase after entering 'solve now.'
1) How do I display the space instead of a dash? 2) Will this automatically move the cursor past the dash when the player types in his guess after entering 'solve now'?
import random
word_list = [
"vampire bite"
]
def game():
wins = 0
losses = 0
print("""
Welcome to hangman. Please enter one letter guess at a time. You have ten chances to make an incorrect guess.
If you think you know the answer, you can enter 'solve now' to enter your guess...but if you make a wrong guess, you lose that game.
If you need help, you can enter 'show vowels' in order to reveal the vowels. This will count as five wrong guesses.
""")
while True:
secret_word = random.choice(word_list)
guess_solution = ''
bad_guesses = []
good_guesses = []
vowels = list('aeiou')
show_vowel = False
while len(bad_guesses) < 10 and len(good_guesses) != len(secret_word):
for i in secret_word:
if i not in good_guesses:
print('-', end='')
elif i not in good_guesses and i == " ":
print(" ", end='')
else:
print(i, end='')
print("")
player_guess = input("Please enter a letter. ")
if player_guess == "quit":
print("Here are your wins and losses: {} wins, {} losses.".format(wins, losses))
print("Goodbye.")
quit()
elif player_guess == "solve now":
guess_solution = input("Enter your word guess. ")
if guess_solution == secret_word:
good_guesses = guess_solution
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
else:
guess_solution = "incorrect"
break
elif player_guess == "show vowels" and show_vowel == False:
for i in secret_word:
if i in vowels:
good_guesses.append(i)
bad_guesses.append(i * 5)
show_vowel = True
elif player_guess == "show vowels" and show_vowel == True:
print("You already had the vowels shown.")
elif len(player_guess) > 1:
print("You can only guess one letter at a time.")
continue
elif player_guess in bad_guesses or player_guess in good_guesses:
print("You already guessed that letter.")
continue
elif not player_guess.isalpha() and player_guess != " ":
print("You can only guess letters, not numbers.")
continue
elif player_guess not in secret_word:
bad_guesses.append(player_guess)
print("{} was a bad guess. You have {} guesses left.".format(player_guess, 10-len(bad_guesses)))
print("")
else:
if i in secret_word:
print("{} was a good guess.".format(player_guess))
print("")
for i in secret_word:
if i == player_guess:
good_guesses.append(player_guess)
if len(good_guesses) == len(secret_word):
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
if len(good_guesses) != len(secret_word) or guess_solution == "incorrect":
print("You didn't guess it. The word was '{}'.".format(secret_word))
losses += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
game()
Thanks.
5 Answers
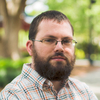
Kenneth Love
Treehouse Guest Teacherprint("-", end=" ")
or print("- ", end="")
?

Iain Simmons
Treehouse Moderator 32,305 PointsDon't you want to always print a space if the character in secret_word
is a space? Because the user isn't expected to guess a space... And I assume if the users enters a space as their guess, it would fail the isalpha()
check and therefore the loop would just continue
...
So:
for i in secret_word:
if i == ' ':
print(' ', end='')
elif i not in good_guesses:
print('-', end='')
else:
print(i, end='')

Gary Gibson
5,011 PointsPlease see my answer to Kenneth.

Iain Simmons
Treehouse Moderator 32,305 PointsI did... but like I said, check for the space first and always print a space if that's what it is, and only if it isn't, do you check whether it is in the good_guesses
or not...

Gary Gibson
5,011 PointsOkay, I'm trying to add print commands and I keep getting this:
Traceback (most recent call last):
File "python", line 31
print(last_word)
^
TabError: inconsistent use of tabs and spaces in indentation
I am not understanding why.

Gary Gibson
5,011 PointsI guess I don't understand because I already showed that I rewrote the code to handle the spaces both in the presentation and the in the solution.
If you run the code, you'll see that the spaces appear where they should and "solve now" allows for spaces to be included in the answer.
I've included several two-or-more-word phrases to illustrate that the code handles them fine now.
import random
word_list = [
"vampire bite",
"New York",
"Frank Castle",
"I come not to bring peace"
]
last_word = []
def get_word():
secret_word = random.choice(word_list)
if secret_word in last_word:
get_word()
return secret_word
def game():
wins = 0
losses = 0
print("""
Welcome to hangman. Please enter one letter guess at a time. You have ten chances to make an incorrect guess.
If you think you know the answer, you can enter 'solve now' to enter your guess...but if you make a wrong guess, you lose that game.
If you need help, you can enter 'show vowels' in order to reveal the vowels. This will count as five wrong guesses.
""")
while True:
secret_word = get_word()
last_word.append(secret_word)
guess_solution = ''
bad_guesses = []
bad_consonants = []
good_guesses = []
vowels = list('aeiou')
show_vowel = False
while len(bad_guesses) < 10 and len(good_guesses) != len(secret_word):
for i in secret_word:
if i not in good_guesses and i != " ":
print('-', end='')
else:
print(i, end='')
if i not in good_guesses and i == ' ':
print(" ", end='')
print("")
player_guess = input("Please enter a letter. ")
if player_guess == "quit":
print("Here are your wins and losses: {} wins, {} losses.".format(wins, losses))
print("Goodbye.")
quit()
elif player_guess == "solve now":
guess_solution = input("Enter your word guess. ")
if guess_solution == secret_word:
good_guesses = guess_solution
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
else:
guess_solution = "incorrect"
break
elif player_guess == "show vowels" and show_vowel == False:
for i in secret_word:
if i in vowels:
good_guesses.append(i)
bad_guesses.append(i * 5)
show_vowel = True
elif player_guess == "show vowels" and show_vowel == True:
print("You already had the vowels shown.")
elif len(player_guess) > 1:
print("You can only guess one letter at a time.")
continue
elif player_guess in good_guesses:
print("You already guessed that letter.")
continue
elif player_guess in bad_consonants:
print("You already guessed that letter.")
print("Your bad guesses include: {}.".format(bad_consonants))
continue
elif not player_guess.isalpha() and player_guess != " ":
print("You can only guess letters, not numbers.")
continue
elif player_guess not in secret_word:
bad_guesses.append(player_guess)
bad_consonants.append(player_guess)
print("{} was a bad guess. You have {} guesses left.".format(player_guess, 10-len(bad_guesses)))
print("You're bad guesses include: {}.".format(str(bad_consonants)))
print("")
else:
if i in secret_word:
print("{} was a good guess.".format(player_guess))
print("")
for i in secret_word:
if i == player_guess:
good_guesses.append(player_guess)
if len(good_guesses) == len(secret_word):
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
if len(good_guesses) != len(secret_word) or guess_solution == "incorrect":
print("You didn't guess it. The word was '{}'.".format(secret_word))
losses += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
game()

Iain Simmons
Treehouse Moderator 32,305 PointsI don't see that in your code, but it's pretty much how it says in the error, don't use both tabs and spaces to indent your code. If you use a good text editor (Sublime Text, Atom, etc) it should highlight spaces and tabs differently when selected, and you may even be able to convert one to the other fairly simply (or do a find and replace with regex).

Iain Simmons
Treehouse Moderator 32,305 PointsYeah it works, but you asked what the "best way" would be, and I just suggested a simpler and I think clearer example of how to run through that logic. But of course, you're free to use whatever works, however it works!

Gary Gibson
5,011 Pointslain, sorry, I didn't understand that you were improving it. I thought you were trying to get it to work. I missed that.

Iain Simmons
Treehouse Moderator 32,305 PointsNo problem! Sorry I didn't make it clear what I was trying to add. Great work on the app in any case!

Gary Gibson
5,011 PointsThank you much! I'm still only a couple months into programming and this has been my biggest piece of reworking yet.
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsSaving throw vs. Intelligence worked this time!
I was having a hard time figuring out how to tell Python what to do when it saw a space in the secret word. But I think I got it now.
This works, but I'm not sure this is the best way to get the desired result ("Pythonic"?) . I tried moving that second if around, but I either get an error about indentation, or I get a dash, then a letter so that each letter throughout the phrase is preceded by a dash like so:
-v-a-m-p-i-r-e- b-i-t-e