Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial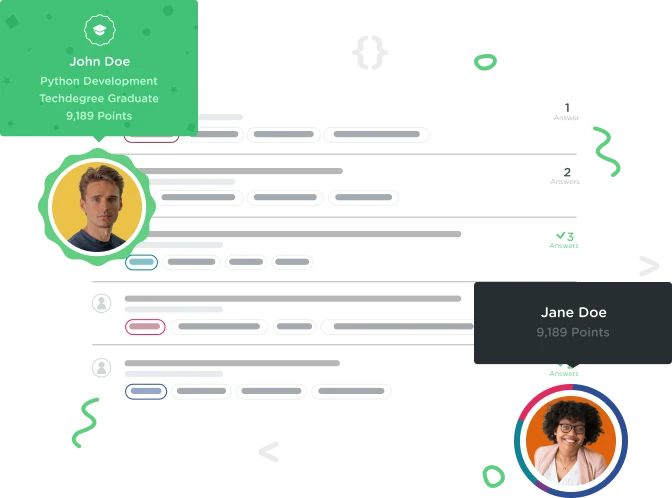
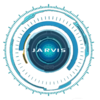
jenyufu
3,311 PointsHow do I make the Username login field also accept logging via the email they signed up for?
I want to make it like most websites where you can sign in with either Username or email.
2 Answers

Orca Z
9,675 PointsIf I'm understanding you right, you wanted to allow the user to type in either their username or email in the edittext on the login screen and sign in to their account. I don't know if Parse.com provides any function doing that but I'm guessing no.
I would suggest you start by checking user's input in the username edittext field and see if it contains "@" symbol.
If it doesn't, simply proceed with normal login process.
If it does contain "@", you will know it's an email input. Set up a query for ParseUser looking for one with that email address and get the username so you can use it to do normal login. Of course if no user with that email is found, just throw out some toast or alert dialog telling user to try again.
And obviously it is necessary to make sure your users' usernames don't include "@" in the first place when they sign up.
Edit: I was thinking about something like this.
// checks if Username field contains "@", mUsername is the text extracted from username edittext.
if(mUsername.contains("@")){
// if it does, what the user typed in the Username EditText should be an email address
// set up query looking for any user with that email address
ParseQuery<ParseUser> emailLoginQuery = ParseUser.getQuery();
emailLoginQuery.whereEqualTo("email", mUsername);
emailLoginQuery.findInBackground(new FindCallback<ParseUser>() {
@Override
public void done(List<ParseUser> list, ParseException e) {
if(list.size() == 0){
// if the result list size is 0, no user with entered email exists.
// Set up an alert dialog or something
}else{
// otherwise there should be 1 user with that email, so we update the mUsername variable with his/her actual username
mUsername = list.get(0).getUsername();
// use this updated mUsername to perform login process
logIn();
}
}
});
}else {
// if mUsername doesn't inlude "@", perform normal login
// logIn() would be a function containing the ParseUser.logInInBackground()... codes
logIn();
}
As of making sure users don't sign up with username including "@", simple if(username.indexOf("@")) or contains("@") should be enough I suppose.
And I didn't even think about checking as username first, I just thought maybe prevent users from being able to create new account with "@" in their usernames .

Douglas Counts
10,060 PointsTo do that, simply send both fields even if one is an empty string. Then check which was submitted on the back end with an if-statement.
jenyufu
3,311 Pointsjenyufu
3,311 Points"And obviously it is necessary to make sure your users' usernames don't include "@" in the first place when they sign up."
So to avoid that, I can just check username first, and then check email if username doesn't work so it will allow for people with "@" in their username to login?
so something like:
if (username.indexOf("@"))
honestly, I'm still pretty confused and help would be much appreciated.
jenyufu
3,311 Pointsjenyufu
3,311 Pointsoh and here is my code to LoginActivity right now
jenyufu
3,311 Pointsjenyufu
3,311 PointsIt worked!!! thanks, I have been trying to solve this problem for days. Here is the code for anyone in the future to look at:
my LoginActivity is a bit different from the one in the tutorial because mine was built with ParseLogin. The main difference being I didn't immediately convert my usernameVariable with .getText().toString(); until way later.