Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial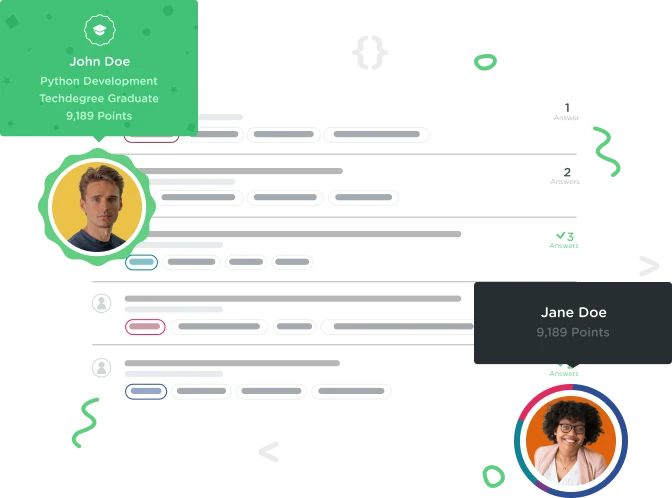

Liam Maclachlan
22,805 PointsHow do I make this jQuery function accept an 'em' measurement instead of just in int 'px' measurement?
I have this code
$('#logo').click(function() {
if ($(window).width > 1000) {
$('nav ul').toggle();
}
});
but I want to use ems instead of pixels. I imagine the pseudo code would look something like this
$('#logo').click(function() {
if ($(window).width > 33em) {
$('nav ul').toggle();
}
});
I feel, right now, that I will need to figure out what the browser is using as 1em and then calculate it... any ideas or more practical ways of doing this?
4 Answers

Roberto Alicata
Courses Plus Student 39,959 PointsTry this code
$('#logo').click(function() {
if ($(window).width() < em(33)) {
$('nav ul').toggle();
}
});
function em(input) {
var emSize = parseFloat($("body").css("font-size"));
return (emSize * input);
}
function px(input) {
var emSize = parseFloat($("body").css("font-size"));
return (Math.floor(input / emSize));
}
remember: when you call the width property you need to add the parenthesis to the end:
$(window).width()

Kyle Daugherty
16,441 PointsThis should work:
$(window).width() / parseFloat($("html").css("font-size"));

Liam Maclachlan
22,805 Pointsclose. I think that is certainly part of what I am looking for :)
var breakPoint = parseFloat(($('html').css('font-size')))*33;
little bit cleaner than my verion now ^_^

Liam Maclachlan
22,805 PointsDon't worry. I had to calculate it but it works perfectly :)
$('#logo').click(function() {
var breakPoint = parseFloat(($('html').css('font-size')))*33;
if (newWidth > $(window).width()) {
$('nav ul').toggle();
}
});
Please post alternatives to this if there is a cleaner way to present/process this code ^_^

Roberto Alicata
Courses Plus Student 39,959 PointsThe jQuery documentation says:
.width( value )
value
Type: String or Number
An integer representing the number of pixels, or an integer along with an optional unit of measure appended (as a string).
so if you want to use em instead of px you need to pass it in a string:
$("div").width("18em");
Liam Maclachlan
22,805 PointsLiam Maclachlan
22,805 PointsOoh. I like that :) I figured out a shorter version but this is great :))
edit: changed my mind. This answer is way more robust. Great answer man. Very clean :)
Roberto Alicata
Courses Plus Student 39,959 PointsRoberto Alicata
Courses Plus Student 39,959 PointsIf you need to convert em to px or vice versa more often in your code , you should create two functions to accomplish these jobs like in my code And it will be more readable when you call $(window).width() < em(33))