Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial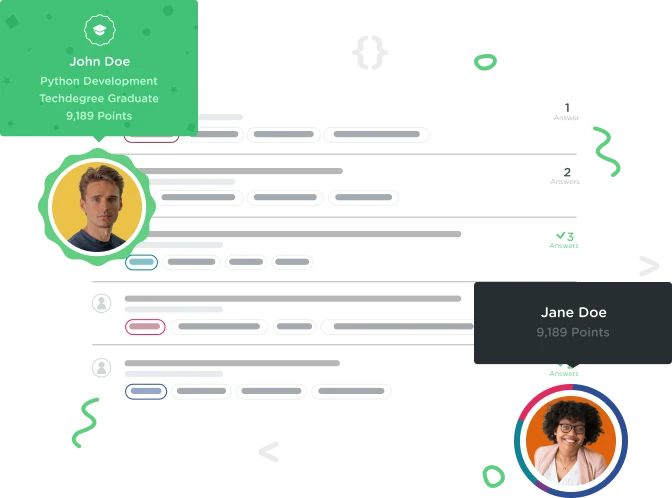
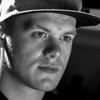
nick schubert
3,535 PointsHow do I make this work? - Python Collections
Hey there, I'm not sure how I complete this challenge. I have an empty dictionary and I have the words split up into lowercase.
Now I have the following questions:
- How do I add the words into the dictionary? Since dicts need a key and a value, how will it add the words without a value?
- How can I then see if there's duplicate words in the dict and add +1 to the value of the word.
I have checked the community for answers and even though I have seen how some people do it, I feel like I lack the knowledge and connect the dots in my head to complete this challenge. Have I perhaps missed some info? Anyway, all help is appreciated! Thanks!
# E.g. word_count("I do not like it Sam I Am") gets back a dictionary like:
# {'i': 2, 'do': 1, 'it': 1, 'sam': 1, 'like': 1, 'not': 1, 'am': 1}
# Lowercase the string to make it easier.
def word_count(argument):
words = argument.lower().split()
dictionary = {}
for word in dictionary:
#TODO: detect if there's duplicate words in the dictionary then...???
1 Answer

andren
28,558 PointsHow do I add the words into the dictionary? Since dicts need a key and a value, how will it add the words without a value?
You aren't supposed to add them without a value, the returned dictionary is supposed to have the key be the word and the value be the number of times it appears. But the value can be changed as your code runs, so it does not need to be correct at the time you insert the word. So when you insert the word you can just set the value as 1 the first time you insert the word, and then add to that value the next time that word comes up.
How can I then see if there's duplicate words in the dict and add +1 to the value of the word.
You can't, a dictionary does not allow duplicate keys, so each word will only have one entry in the dictionary.
The way around this is to do both of those steps at once. Deal with detecting duplicates while you add the words to the dictionary. While there are more than one way of solving this challenge by far the simplest is to do something along these lines:
- Create a loop that pulls out each word from the
words
variable you have created - Check if the pulled out word currently exists in the dictionary
- If it does not exist then add it and set its value to 1.
- If it does exist then take the current value stored in the dictionary and increase it by 1.
After that loop finishes you will end up with a dictionary that has all of the words inserted and has accurate values associated with each one. Which means you don't have to do anything more with the dictionary beyond returning it.
nick schubert
3,535 Pointsnick schubert
3,535 PointsThank you so much! Thanks to your explanation everything makes sense and I managed to complete the challenge.
Incase anyone else runs into the same problem as me, here's the code I completed the challenge with: