Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial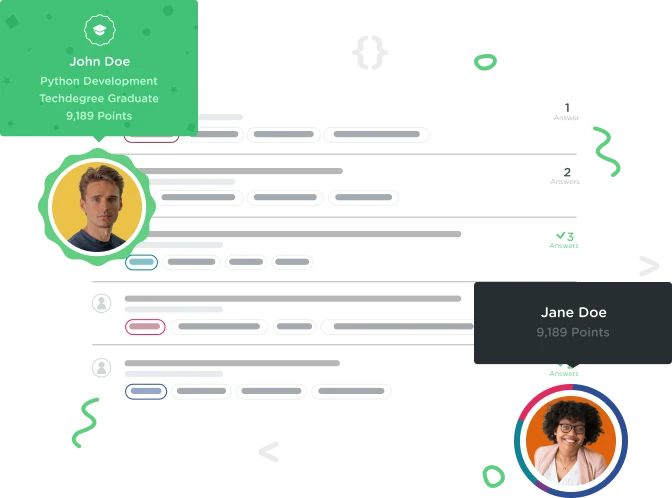
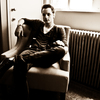
Paul Hubbard
5,818 PointsHow do I pass an array into a function parameter?
I've been trying this challenge over and over again, and I'm trying to figure out how to pass an array as a function parameter, and then call the array inside the function.
Please Help!
<!DOCTYPE html>
<html lang="en">
<head>
<title>JavaScript Foundations: Functions</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Functions: Return Values</h2>
<script>
</script>
</body>
</html>
1 Answer
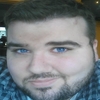
Marcus Parsons
15,719 PointsHey Paul Hubbard,
When you're passing in arguments to a function, an argument can be pretty much anything. It can be a string, number, object/array, another function, etc. When it tells you that you're passing in an array, it is more to let you know what this function will do rather than what to do with that argument. All you have to do is check the type of the argument passed in and make sure it isn't any of the things listed. You can't check to see if something is an array, specifically, because an array is the same thing as an object. But, you can check if it's the other types like so:
function arrayCounter (arr) {
//Check to see if the passed in argument is a string, number, or undefined
if (typeof arr == "string" || typeof arr == "number" || typeof arr == "undefined") {
//If so, return 0, which breaks out of the function
return 0;
}
else {
//If it's not any of those things, it is of type "object" so it can be an array which is the same as an object
return arr.length;
}
}