Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial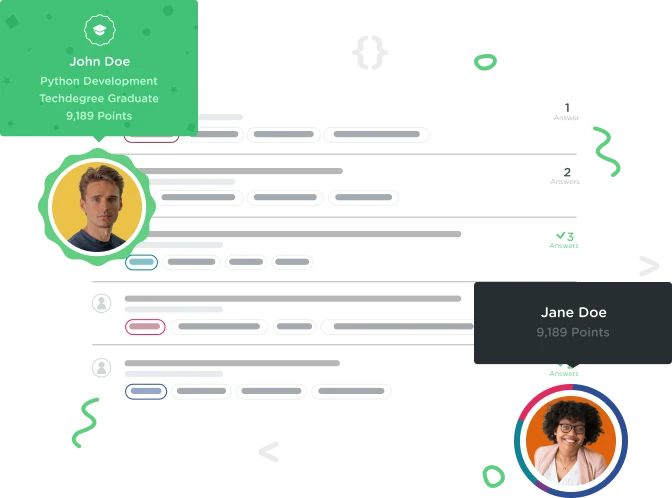

Michael McKenna
3,451 PointsHow do I pause my sound playing in the background in my app?
So I press a button and my app goes to the credits where Concerning Hobbits starts playing. I want to have the music stop (or in this case, it would be mPlayer.pause() ) when the user presses the back button on their phone. I know how I would do this if I was to add a button on the screen to go back to the Main Activity, but how do I make the music pause when the back button on the phone is pressed?
10 Answers
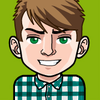
Harry James
14,780 PointsHey Michael!
All you need to do is create a onBackPressed()
listener instead! Then, in the method, just put that mPlayer.pause()
line in!
Hope it helps and, if you have any more questions, give me a shout :)

Michael McKenna
3,451 PointsWow you are awesome!

Michael McKenna
3,451 PointsHowdy Harry,
Thank you for your answer. I tried using what you said in my MainActivity and the activity I put below, but neither would do the trick. The music kept playing whenever I pressed back and went to my main menu. Any ideas? If you need any more information just let me know!
public class DisplayMessageActivity extends ActionBarActivity {
private TextView mTextView;
private String mAnswer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display_message);
mTextView = (TextView) findViewById(R.id.textView);
Intent intent = getIntent();
String message = intent.getStringExtra("answer");
mTextView.setText(message);
// Get the message from the intent
Intent intent2 = getIntent();
mAnswer = intent2.getStringExtra("answer");
mTextView.setText(mAnswer);
Intent intent3 = getIntent();
String credits = intent3.getStringExtra("answer");
mTextView.setText(credits);
}
public void onBackPressed() {
MediaPlayer mp = new MediaPlayer();
mp.stop();
}
}
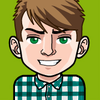
Harry James
14,780 PointsHey Michael!
Is there a reason you're creating a new MediaPlayer object? What you're doing here is creating a brand new MediaPlayer and then stopping the audio from that MediaPlayer (However, it was never set to play any media!)

Michael McKenna
3,451 PointsI took your advice Harry and made some changes. However, I am getting a null pointer exception error and, in addition, I am having trouble calling my MediaPlayer from my MainActivity into my DisplayMessageActivity. If I move out the Media Player in MainActivity and make it public, I can call it in DisplayMessageActivity by doing new MainActivity.mPlayer.stop(). However, at this point I get the null pointer exception so I moved the MediaPlayer back into the oncreate(saved instancestate). When I do this, I can't call my MediaPlayer! Haha, so I am at a loss right now.
My MainActivity is:
package com.calcwork.michael.math151hw.UI;
import android.content.Intent;
import android.media.MediaPlayer;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuInflater;
import android.view.View;
import android.widget.Button;
import com.calcwork.michael.math151hw.R;
public class MainActivity extends ActionBarActivity {
private Button mButton;
private Button mButton2;
private Button mButton3;
private Button mButton4;
private Button mButton5;
private Button mButton6;
private Button mButton7;
private Button mButton8;
private Button mButton9;
private Button mButton10;
private Button mButton11;
private Button mButton12;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
mButton = (Button)findViewById(R.id.button);
mButton2 = (Button)findViewById(R.id.button2);
mButton3 = (Button)findViewById(R.id.button3);
mButton4 = (Button)findViewById(R.id.button5);
mButton5 = (Button)findViewById(R.id.button6);
mButton6 = (Button)findViewById(R.id.button7);
mButton7 = (Button)findViewById(R.id.button8);
mButton8 = (Button)findViewById(R.id.button9);
mButton9 = (Button)findViewById(R.id.button10);
mButton10 = (Button)findViewById(R.id.button11);
mButton11 = (Button)findViewById(R.id.button12);
final MediaPlayer mPlayer = MediaPlayer.create(MainActivity.this, R.raw.concerning_hobbits);
mButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String answer = "2x";
startButton(answer);
}
});
mButton2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String answer2 = "2e^2x";
startButton2(answer2);
}
});
mButton3.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
String answer3 = "Created by Michael McKenna";
mPlayer.start();
startButton3(answer3);
}
});
}
public void startButton(String answer) {
Intent intent = new Intent(this, DisplayMessageActivity.class);
intent.putExtra("answer",answer);
startActivity(intent);
}
private void startButton2(String answer2) {
Intent intent2 = new Intent(this, DisplayMessageActivity.class);
intent2.putExtra("answer",answer2);
startActivity(intent2);
}
public void startButton3(String answer3) {
Intent intent3 = new Intent(this, DisplayMessageActivity.class);
intent3.putExtra("answer",answer3);
startActivity(intent3);
}
}
My DisplayMessageActivity is (with my problem being at the bottom of my code):
package com.calcwork.michael.math151hw.UI;
import android.content.Context;
import android.content.Intent;
import android.media.AudioManager;
import android.media.MediaPlayer;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.widget.TextView;
import com.calcwork.michael.math151hw.R;
public class DisplayMessageActivity extends ActionBarActivity {
private MainActivity DisplayMessageActivity;
private TextView mTextView;
private String mAnswer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display_message);
mTextView = (TextView) findViewById(R.id.textView);
Intent intent = getIntent();
String message = intent.getStringExtra("answer");
mTextView.setText(message);
// Get the message from the intent
Intent intent2 = getIntent();
mAnswer = intent2.getStringExtra("answer");
mTextView.setText(mAnswer);
Intent intent3 = getIntent();
String credits = intent3.getStringExtra("answer");
mTextView.setText(credits);
}
public void onBackPressed() {
super.onBackPressed();
new MainActivity().onCreate().mPlayer.stop();
}
}
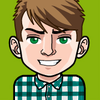
Harry James
14,780 PointsI see you're trying to stop the MediaPlayer from a different activity than it was created in! This is a bit more complicated but there's a StackOverflow page on it here if you're interested.
There's a few ways to go about it but, I'd highly recommend you both create and stop the MediaPlayer from the same activity if that is possible for you. If you start audio from one activity, it will still carry on to the next.
Hope it helps and, if you're having trouble with any of this, give me a shout and I'll try my best to help out :)

Michael McKenna
3,451 PointsI appreciate your help through this Harry. I'll take a look at the link!
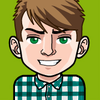
Harry James
14,780 PointsOh! I just had an idea whilst I was in the bath as well!
Perhaps you could initialize the media player in a separate methods on your main activity. Like this:
protected void playMusic() {
mPlayer.start();
}
protected void StopMusic() {
mPlayer.stop();
}
As the methods are protected, they're accessible from anywhere within your package/subclasses!
I haven't tested this yet though and perhaps it might not work as the activity may be classed as "not running" but, try it out and see if it does because if it works, it'll be a lot easier than some of the things on that StackOverflow page!
If you haven't got around to testing it before me, I'll give it a go late tomorrow (I have school before that) and let you know how that goes. Edit: Apologies! I have to revise for an exam I have tomorrow so will take a look at this tomorrow (Thursday) instead!
Hope it helps! :)

Michael McKenna
3,451 PointsNo rush at all Harry! I am taking class engineering classes at Texas A&M and I totally understand the limitations of time constraints ;).
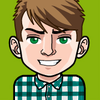
Harry James
14,780 PointsOkay. After some testing, I've found out that once you create a MediaPlayer, go to an activity and return, the MediaPlayer will lose its value.
I'm not sure if there's a way you can pass the value of that MediaPlayer to the 2nd activity and back again as of yet but will try to see if there's anything.
Edit: I can't seem to find a way to do this sadly. I've spent a long time looking but to no avail. Sorry I'm unable to help :'(

Michael McKenna
3,451 PointsI'll just find a way to keep everything in the same activity. Thanks again Harry!
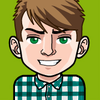
Harry James
14,780 PointsI've heard you can use Services to hold these values but, these haven't yet been taught in the courses and I have never tried them before.
What is it that you are actually trying to do? I might be able to better help you/find the best method of doing this if you let me know what it is (e.g: Are you just trying to make music play across all screens of your app and have a button on all screens allowing you to stop it).

Michael McKenna
3,451 PointsI just placed a button saying (Back to Main Menu) and it stop the music and brings the user back to the main menu. However, when I declare the media player DisplayMessageActivity, it plays the music for every button. My goal is to just have the music play when the user pressed "Credits" while in the MainActivity (hasn't changed; posted above).
package com.calcwork.michael.math151hw.UI;
import android.content.Intent;
import android.media.MediaPlayer;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import com.calcwork.michael.math151hw.R;
public class DisplayMessageActivity extends ActionBarActivity {
private TextView mTextView;
private String mAnswer;
private Button mMainMenu;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_display_message);
mTextView = (TextView) findViewById(R.id.textView);
mMainMenu = (Button)findViewById(R.id.mainMenu);
final MediaPlayer mPlayer = MediaPlayer.create(DisplayMessageActivity.this, R.raw.concerning_hobbits);
Intent intent = getIntent();
String message = intent.getStringExtra("answer");
mTextView.setText(message);
// Get the message from the intent
Intent intent2 = getIntent();
mAnswer = intent2.getStringExtra("answer");
mTextView.setText(mAnswer);
Intent intent3 = getIntent();
String credits = intent3.getStringExtra("answer");
mPlayer.start();
mTextView.setText(credits);
mMainMenu.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
mPlayer.stop();
ButtonReturn();
}
});
}
public void ButtonReturn() {
Intent intent4 = new Intent(this, MainActivity.class);
startActivity(intent4);
}
}
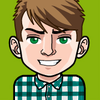
Harry James
14,780 PointsYes, I believe you would have to use a service for this because it seems as though when you leave an activity, the MediaPlayer loses its value on return to the activity...
Sorry I wasn't able to help you out here but, I wish you the best of luck on getting it working :)
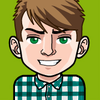
Harry James
14,780 PointsI managed to get it working Michael!
It turns out when I was testing, my button was broken all along so that was causing the error rather than the MediaPlayer and also I forgot to release the MediaPlayer which was keeping the broken code in memory.
Anyhow, here is the code I worked to get it sorted:
MainActivity.java
public class MainActivity extends ActionBarActivity {
// Create the MediaPlayer so that we can access it anywhere in our package.
protected static MediaPlayer mPlayer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Here is our two buttons used for testing.
final Button playButton = (Button) findViewById(R.id.buttonPlay);
final Button newActivityButton = (Button) findViewById(R.id.buttonNewActivity);
playButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View v) {
// If the MediaPlayer hasn't been created yet:
if (mPlayer == null) {
// Initialize our MediaPlayer with our audio file (Replace myaudio).
mPlayer = MediaPlayer.create(MainActivity.this, R.raw.myaudio);
}
// Play the music player.
mPlayer.start();
}
});
newActivityButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Go to MainActivity2.
Intent intent = new Intent(MainActivity.this, MainActivity2.class);
startActivity(intent);
}
});
}
}
MainActivity2.java
public class MainActivity2 extends ActionBarActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main_activity2);
// Here is our stop button used for testing.
final Button stopMusicButton = (Button) findViewById(R.id.buttonStop);
stopMusicButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Stop the MediaPlayer and release it from memory.
MainActivity.mPlayer.stop();
MainActivity.mPlayer.release();
MainActivity.mPlayer = null;
}
});
}
}
activity_main.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingBottom="@dimen/activity_vertical_margin"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
tools:context=".MainActivity">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="PLAY MUSIC"
android:id="@+id/buttonPlay"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginTop="200dp" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="NEW ACTIVITY"
android:id="@+id/buttonNewActivity"
android:layout_below="@+id/buttonPlay"
android:layout_centerHorizontal="true" />
</RelativeLayout>
activity_main_activity2.xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent"
android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context="com.harryjamesuk.testapp2.MainActivity2">
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="STOP MUSIC"
android:id="@+id/buttonStop"
android:layout_centerHorizontal="true"
android:layout_centerVertical="true" />
</RelativeLayout>
Or if you prefer, my Project Files for Android Studio can be found here. There is instructions in the README.txt on how to import the project into your Android Studio.
Hope it helps!