Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial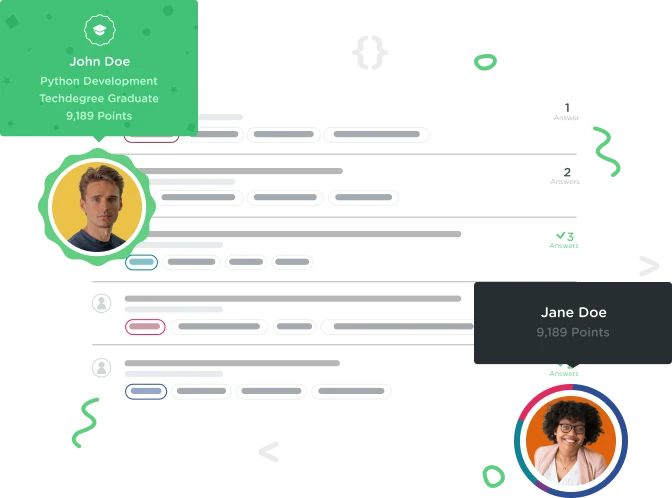

Gary Gibson
5,011 PointsHow do I prevent the code from using the same randomly chosen word it just used for my game?
I've tried, but can't prevent the same random word from being used twice.
I've tried placing a holder for the last word and then using a get_word() function to exclude random words that are the same as the last word.
import random
word_list = [
"vampire bite",
"New York",
"Frank Castle",
]
last_word = []
def get_word():
secret_word = random.choice(word_list)
if secret_word in last_word:
get_word()
return secret_word
def game():
wins = 0
losses = 0
print("""
Welcome to hangman. Please enter one letter guess at a time. You have ten chances to make an incorrect guess.
If you think you know the answer, you can enter 'solve now' to enter your guess...but if you make a wrong guess, you lose that game.
If you need help, you can enter 'show vowels' in order to reveal the vowels. This will count as five wrong guesses.
""")
while True:
secret_word = get_word()
guess_solution = ''
bad_guesses = []
bad_consonants = []
good_guesses = []
vowels = list('aeiou')
show_vowel = False
while len(bad_guesses) < 10 and len(good_guesses) != len(secret_word):
for i in secret_word:
if i not in good_guesses and i != " ":
print('-', end='')
else:
print(i, end='')
if i not in good_guesses and i == ' ':
print(" ", end='')
print("")
print(last_word)
print("")
player_guess = input("Please enter a letter. ")
if player_guess == "quit":
print("Here are your wins and losses: {} wins, {} losses.".format(wins, losses))
print("Goodbye.")
quit()
elif player_guess == "solve now":
guess_solution = input("Enter your word guess. ")
if guess_solution == secret_word:
good_guesses = guess_solution
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
else:
guess_solution = "incorrect"
break
elif player_guess == "show vowels" and show_vowel == False:
for i in secret_word:
if i in vowels:
good_guesses.append(i)
bad_guesses.append(i * 5)
show_vowel = True
elif player_guess == "show vowels" and show_vowel == True:
print("You already had the vowels shown.")
elif len(player_guess) > 1:
print("You can only guess one letter at a time.")
continue
elif player_guess in good_guesses:
print("You already guessed that letter.")
continue
elif player_guess in bad_consonants:
print("You already guessed that letter.")
print("You're bad guesses include: {}.".format(bad_consonants))
continue
elif not player_guess.isalpha() and player_guess != " ":
print("You can only guess letters, not numbers.")
continue
elif player_guess not in secret_word:
bad_guesses.append(player_guess)
bad_consonants.append(player_guess)
print("{} was a bad guess. You have {} guesses left.".format(player_guess, 10-len(bad_guesses)))
print("You're bad guesses include: {}.".format(bad_consonants))
print("")
else:
if i in secret_word:
print("{} was a good guess.".format(player_guess))
print("")
for i in secret_word:
if i == player_guess:
good_guesses.append(player_guess)
if len(good_guesses) == len(secret_word):
print("You got it! {}.".format(secret_word))
wins += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
if len(good_guesses) != len(secret_word) or guess_solution == "incorrect":
print("You didn't guess it. The word was '{}'.".format(secret_word))
losses += 1
print("{} wins, {} losses.".format(wins, losses))
print("")
last_word.append(secret_word)
game()
1 Answer
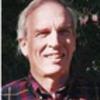
jcorum
71,830 PointsWhen you only have three words in your list the chances of getting the same word twice in a row are very high. The best way to solve the problem is to make you list quite a bit longer. As in the video, he had around 9 or 10 fruits.
Gary Gibson
5,011 PointsGary Gibson
5,011 PointsI only have three words in there as a test to the solution I wrote (which doesn't work!)
I had ten words in there and still found I was getting the same word twice a little too often for my liking. So I'm just seeing if there's a way to prevent that. It also helps me understand what's really going on a bit better to play around with attempts like this.